Notebook¶
A Coronal Mass Ejection (CME) throws magnetic flux and plasma from the Sun into interplanetary space. These eruptions are actually related to solar flares – in fact, CMEs and solar flares are considered “a single magnetically driven event” (Webb & Howard 2012), wherein a flare unassociated with a CME is called a confined or compact flare.
In general, the more energetic a flare, the more likely it is to be associated with a CME (Yashiro et al. 2005) – but this is not, by any means, a rule. For example, Sun et al. (2015) found that the largest active region in the last 24 years, shown below, produced 6 X-class flares but not a single observed CME.
In this notebook, we will be predicting whether or not a flaring active region will also emit a CME using a machine learning algorithm from the scikit-learn package called Support Vector Machine.
The analysis that follows is published in Bobra & Ilonidis, 2016, Astrophysical Journal, 821, 127. If you use any of this code, we ask that you cite Bobra & Ilonidis (2016).
Here is a video that explains the difference between a flare and a CME:
from IPython.display import YouTubeVideo
YouTubeVideo("TWjtYSRlOUI")
To do this analysis, we’ll look at every active region observed by the Helioseismic and Magnetic Imager instrument on NASA’s Solar Dynamics Observatory (SDO) satellite over the last eight years. Each active region is characterized by a bunch of features. These features describe the magnetic field at the solar surface. One feature, for example, is the total energy contained within an active region. Another is the total flux through an active region. We have 18 features, all of which are calculated every 12 minutes throughout an active region’s lifetime. See Bobra et al., 2014 for more information on how we calculate these features.
We’ll then ascribe each active region to one of two classes:
The positive class contains flaring active regions that did produce a CME.
The negative class contains flaring active regions that did not produce a CME.
First, we’ll import some modules.
import numpy as np
import matplotlib.pylab as plt
import matplotlib.mlab as mlab
import pandas as pd
import scipy.stats
import requests
import urllib
import json
from datetime import datetime as dt_obj
from datetime import timedelta
from sklearn import svm
from sklearn.model_selection import StratifiedKFold
from sunpy.time import TimeRange
import sunpy.instr.goes
import lime
import lime.lime_tabular
pd.set_option('display.max_rows', 500)
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
Now we’ll gather the data. The data come from three different places:
CME data from SOHO/LASCO and STEREO/SECCHI coronographs, which can be accesed from the DONKI database at NASA Goddard. This tells us if an active region has produced a CME or not.
Flare data from the GOES flare catalog at NOAA, which can be accessed with the
sunpy.instr.goes.get_event_list()
function. This tells us if an active region produced a flare or not.Active region data from the Solar Dynamics Observatory’s Heliosesmic and Magnetic Imager instrument, which can be accessed from the JSOC database via a JSON API. This gives us the features characterizing each active region.
Step 1: Gathering data for the positive class¶
Let’s first query the DONKI database to get the data associated with the positive class. Be forewarned: there’s a lot of data cleaning involved with building the positive class.
# request the data
baseurl = "https://kauai.ccmc.gsfc.nasa.gov/DONKI/WS/get/FLR?"
t_start = "2010-05-01"
t_end = "2018-04-01"
url = baseurl+"startDate="+t_start+"&endDate="+t_end
# if there's no response at this time, print warning
response = requests.get(url)
if response.status_code != 200:
print('cannot successfully get an http response')
# read the data
print("Getting data from", url)
df = pd.read_json(url)
# select flares associated with a linked event (SEP or CME), and
# select only M or X-class flares
events_list = df.loc[df['classType'].str.contains(
"M|X") & ~df['linkedEvents'].isnull()]
# drop all rows that don't satisfy the above conditions
events_list = events_list.reset_index(drop=True)
Getting data from https://kauai.ccmc.gsfc.nasa.gov/DONKI/WS/get/FLR?startDate=2010-05-01&endDate=2018-04-01
# drop the rows that aren't linked to CME events
for i in range(events_list.shape[0]):
value = events_list.loc[i]['linkedEvents'][0]['activityID']
if not "CME" in value:
print(value, "not a CME, dropping row")
events_list = events_list.drop([i])
events_list = events_list.reset_index(drop=True)
2011-08-09T08:40:00-SEP-001 not a CME, dropping row
2011-09-07T06:00:00-SEP-001 not a CME, dropping row
2011-09-24T11:50:00-SEP-001 not a CME, dropping row
2011-09-24T20:45:00-SEP-001 not a CME, dropping row
2012-07-09T01:20:00-SEP-001 not a CME, dropping row
2013-06-21T06:09:00-SEP-001 not a CME, dropping row
2015-03-06T19:20:00-SEP-001 not a CME, dropping row
2015-06-18T09:25:00-SEP-001 not a CME, dropping row
2015-06-21T20:35:00-SEP-001 not a CME, dropping row
2015-06-21T20:35:00-SEP-001 not a CME, dropping row
Convert the peakTime
column in the events_list
dataframe from a string into a datetime object:
def parse_tai_string(tstr):
year = int(tstr[:4])
month = int(tstr[5:7])
day = int(tstr[8:10])
hour = int(tstr[11:13])
minute = int(tstr[14:16])
return dt_obj(year, month, day, hour, minute)
for i in range(events_list.shape[0]):
events_list['peakTime'].iloc[i] = parse_tai_string(
events_list['peakTime'].iloc[i])
Check for Case 1: In this case, the CME and flare exist but NOAA active region number does not exist in the DONKI database.
# Case 1: CME and Flare exist but NOAA active region number does not exist in DONKI database
number_of_donki_mistakes = 0 # count the number of DONKI mistakes
# create an empty array to hold row numbers to drop at the end
event_list_drops = []
for i in range(events_list.shape[0]):
if (np.isnan(events_list.loc[i]['activeRegionNum'])):
time = events_list['peakTime'].iloc[i]
time_range = TimeRange(time, time)
listofresults = sunpy.instr.goes.get_goes_event_list(time_range, 'M1')
if (listofresults[0]['noaa_active_region'] == 0):
print(events_list.loc[i]['activeRegionNum'], events_list.loc[i]
['classType'], "has no match in the GOES flare database ; dropping row.")
event_list_drops.append(i)
number_of_donki_mistakes += 1
continue
else:
print("Missing NOAA number:", events_list['activeRegionNum'].iloc[i], events_list['classType'].iloc[i],
events_list['peakTime'].iloc[i], "should be", listofresults[0]['noaa_active_region'], "; changing now.")
events_list['activeRegionNum'].iloc[i] = listofresults[0]['noaa_active_region']
number_of_donki_mistakes += 1
# Drop the rows for which there is no active region number in both the DONKI and GOES flare databases
events_list = events_list.drop(event_list_drops)
events_list = events_list.reset_index(drop=True)
print('There are', number_of_donki_mistakes, 'DONKI mistakes so far.')
Missing NOAA number: nan X1.4 2011-09-22 11:01:00 should be 11302 ; changing now.
Missing NOAA number: nan X1.3 2012-03-07 01:14:00 should be 11430 ; changing now.
Missing NOAA number: nan M6.3 2012-03-09 03:53:00 should be 11429 ; changing now.
Missing NOAA number: nan M5.1 2012-05-17 01:47:00 should be 11476 ; changing now.
Missing NOAA number: nan X1.1 2012-07-06 23:08:00 should be 11515 ; changing now.
Missing NOAA number: nan M6.2 2012-07-28 20:56:00 should be 11532 ; changing now.
Missing NOAA number: nan M1.7 2012-11-08 02:23:00 should be 11611 ; changing now.
Missing NOAA number: nan M1.2 2013-03-15 06:58:00 should be 11692 ; changing now.
Missing NOAA number: nan X1.6 2013-05-13 02:17:00 should be 11748 ; changing now.
Missing NOAA number: nan X2.8 2013-05-13 16:05:00 should be 11748 ; changing now.
Missing NOAA number: nan X3.2 2013-05-14 01:11:00 should be 11748 ; changing now.
Missing NOAA number: nan X1.2 2013-05-15 01:48:00 should be 11748 ; changing now.
Missing NOAA number: nan M5.0 2013-05-22 13:38:00 should be 11745 ; changing now.
nan M1.0 has no match in the GOES flare database ; dropping row.
nan M1.1 has no match in the GOES flare database ; dropping row.
Missing NOAA number: nan X4.9 2014-02-25 00:49:00 should be 11990 ; changing now.
nan M1.4 has no match in the GOES flare database ; dropping row.
nan M2.2 has no match in the GOES flare database ; dropping row.
Missing NOAA number: nan M3.0 2015-03-06 04:57:00 should be 12297 ; changing now.
Missing NOAA number: nan M1.5 2015-03-06 08:15:00 should be 12297 ; changing now.
nan M2.2 has no match in the GOES flare database ; dropping row.
Missing NOAA number: nan M2.8 2015-12-21 01:03:00 should be 12472 ; changing now.
Missing NOAA number: nan M1.1 2015-12-21 10:19:00 should be 12472 ; changing now.
There are 23 DONKI mistakes so far.
Now we grab all the data from the GOES database in preparation for checking Cases 2 and 3.
# Grab all the data from the GOES database
time_range = TimeRange(t_start, t_end)
listofresults = sunpy.instr.goes.get_goes_event_list(time_range, 'M1')
print('Grabbed all the GOES data; there are', len(listofresults), 'events.')
Grabbed all the GOES data; there are 793 events.
Check for Case 2: In this case, the NOAA active region number is wrong in the DONKI database.
# Case 2: NOAA active region number is wrong in DONKI database
# collect all the peak flares times in the NOAA database
peak_times_noaa = [item["peak_time"] for item in listofresults]
for i in range(events_list.shape[0]):
# check if a particular DONKI flare peak time is also in the NOAA database
peak_time_donki = events_list['peakTime'].iloc[i]
if peak_time_donki in peak_times_noaa:
index = peak_times_noaa.index(peak_time_donki)
else:
continue
# ignore NOAA active region numbers equal to zero
if (listofresults[index]['noaa_active_region'] == 0):
continue
# if yes, check if the DONKI and NOAA active region numbers match up for this peak time
# if they don't, flag this peak time and replace the DONKI number with the NOAA number
if (listofresults[index]['noaa_active_region'] != int(events_list['activeRegionNum'].iloc[i])):
print('Messed up NOAA number:', int(events_list['activeRegionNum'].iloc[i]), events_list['classType'].iloc[i],
events_list['peakTime'].iloc[i], "should be", listofresults[index]['noaa_active_region'], "; changing now.")
events_list['activeRegionNum'].iloc[i] = listofresults[index]['noaa_active_region']
number_of_donki_mistakes += 1
print('There are', number_of_donki_mistakes, 'DONKI mistakes so far.')
Messed up NOAA number: 11943 X1.2 2014-01-07 18:32:00 should be 11944 ; changing now.
Messed up NOAA number: 12051 M1.2 2014-05-07 16:29:00 should be 12055 ; changing now.
Messed up NOAA number: 12160 M1.4 2014-07-01 11:23:00 should be 12106 ; changing now.
Messed up NOAA number: 12282 M2.4 2015-02-09 23:35:00 should be 12280 ; changing now.
There are 27 DONKI mistakes so far.
Check for Case 3: In this case, the flare peak time is wrong in the DONKI database.
# Case 3: The flare peak time is wrong in the DONKI database.
# create an empty array to hold row numbers to drop at the end
event_list_drops = []
active_region_numbers_noaa = [item["noaa_active_region"]
for item in listofresults]
flare_classes_noaa = [item["goes_class"] for item in listofresults]
for i in range(events_list.shape[0]):
# check if a particular DONKI flare peak time is also in the NOAA database
peak_time_donki = events_list['peakTime'].iloc[i]
if not peak_time_donki in peak_times_noaa:
active_region_number_donki = int(
events_list['activeRegionNum'].iloc[i])
flare_class_donki = events_list['classType'].iloc[i]
flare_class_indices = [i for i, x in enumerate(
flare_classes_noaa) if x == flare_class_donki]
active_region_indices = [i for i, x in enumerate(
active_region_numbers_noaa) if x == active_region_number_donki]
common_indices = list(
set(flare_class_indices).intersection(active_region_indices))
if common_indices:
print("Messed up time:", int(events_list['activeRegionNum'].iloc[i]), events_list['classType'].iloc[i],
events_list['peakTime'].iloc[i], "should be", peak_times_noaa[common_indices[0]], "; changing now.")
events_list['peakTime'].iloc[i] = peak_times_noaa[common_indices[0]]
number_of_donki_mistakes += 1
if not common_indices:
print("DONKI flare peak time",
events_list['peakTime'].iloc[i], "has no match; dropping row.")
event_list_drops.append(i)
number_of_donki_mistakes += 1
# Drop the rows for which the NOAA active region number and flare class associated with
# the messed-up flare peak time in the DONKI database has no match in the GOES flare database
events_list = events_list.drop(event_list_drops)
events_list = events_list.reset_index(drop=True)
# Create a list of corrected flare peak times
peak_times_donki = [events_list['peakTime'].iloc[i]
for i in range(events_list.shape[0])]
print('There are', number_of_donki_mistakes, 'DONKI mistakes so far.')
Messed up time: 11158 X2.2 2011-02-15 01:56:00 should be 2011-02-15T01:56:00.000 ; changing now.
Messed up time: 11302 X1.4 2011-09-22 11:01:00 should be 2011-09-22T11:01:00.000 ; changing now.
Messed up time: 11429 X1.1 2012-03-05 04:05:00 should be 2012-03-05T04:09:00.000 ; changing now.
Messed up time: 11429 X5.4 2012-03-07 00:24:00 should be 2012-03-07T00:24:00.000 ; changing now.
Messed up time: 11430 X1.3 2012-03-07 01:14:00 should be 2012-03-07T01:14:00.000 ; changing now.
DONKI flare peak time 2012-03-10 17:27:00 has no match; dropping row.
Messed up time: 11476 M5.1 2012-05-17 01:47:00 should be 2012-05-17T01:47:00.000 ; changing now.
DONKI flare peak time 2013-03-15 06:58:00 has no match; dropping row.
Messed up time: 11719 M6.5 2013-04-11 07:16:00 should be 2013-04-11T07:16:00.000 ; changing now.
Messed up time: 11748 X3.2 2013-05-14 01:11:00 should be 2013-05-14T01:11:00.000 ; changing now.
Messed up time: 11748 X1.2 2013-05-15 01:48:00 should be 2013-05-15T01:48:00.000 ; changing now.
Messed up time: 11745 M5.0 2013-05-22 13:38:00 should be 2013-05-22T13:32:00.000 ; changing now.
Messed up time: 11877 M9.3 2013-10-24 00:30:00 should be 2013-10-24T00:30:00.000 ; changing now.
Messed up time: 11882 X1.7 2013-10-25 08:01:00 should be 2013-10-25T08:01:00.000 ; changing now.
DONKI flare peak time 2014-02-09 16:14:00 has no match; dropping row.
Messed up time: 12035 X1.3 2014-04-25 00:27:00 should be 2014-04-25T00:27:00.000 ; changing now.
Messed up time: 12087 X2.2 2014-06-10 11:42:00 should be 2014-06-10T11:42:00.000 ; changing now.
Messed up time: 12127 M1.5 2014-08-01 18:12:00 should be 2014-08-01T18:13:00.000 ; changing now.
Messed up time: 12146 M2.0 2014-08-25 15:10:00 should be 2014-08-25T15:11:00.000 ; changing now.
DONKI flare peak time 2014-09-03 13:53:00 has no match; dropping row.
DONKI flare peak time 2014-09-09 00:28:00 has no match; dropping row.
Messed up time: 12172 M2.3 2014-09-23 23:15:00 should be 2014-09-23T23:16:00.000 ; changing now.
Messed up time: 12192 M4.0 2014-10-24 07:48:00 should be 2014-10-24T07:48:00.000 ; changing now.
Messed up time: 12205 M2.6 2014-11-04 08:38:00 should be 2014-11-04T08:38:00.000 ; changing now.
Messed up time: 12241 M1.1 2014-12-17 01:50:00 should be 2014-12-17T01:50:00.000 ; changing now.
Messed up time: 12242 M8.7 2014-12-17 04:51:00 should be 2014-12-17T04:51:00.000 ; changing now.
Messed up time: 12242 X1.8 2014-12-20 00:24:00 should be 2014-12-20T00:28:00.000 ; changing now.
Messed up time: 12297 M3.0 2015-03-06 04:57:00 should be 2015-03-06T04:57:00.000 ; changing now.
Messed up time: 12297 M5.1 2015-03-10 03:24:00 should be 2015-03-10T03:24:00.000 ; changing now.
Messed up time: 12321 M1.1 2015-04-23 10:07:00 should be 2015-04-12T09:50:00.000 ; changing now.
Messed up time: 12371 M2.6 2015-06-21 02:36:00 should be 2015-06-21T02:36:00.000 ; changing now.
Messed up time: 12403 M1.4 2015-08-21 09:48:00 should be 2015-08-21T09:48:00.000 ; changing now.
Messed up time: 12403 M1.2 2015-08-22 06:49:00 should be 2015-08-21T02:18:00.000 ; changing now.
Messed up time: 12423 M3.6 2015-09-28 03:55:00 should be 2015-09-28T03:55:00.000 ; changing now.
Messed up time: 12445 M1.9 2015-11-04 03:25:00 should be 2015-11-04T03:26:00.000 ; changing now.
Messed up time: 12472 M2.8 2015-12-21 01:03:00 should be 2015-12-21T01:03:00.000 ; changing now.
Messed up time: 12472 M1.1 2015-12-21 10:19:00 should be 2015-12-21T10:19:00.000 ; changing now.
Messed up time: 12473 M4.7 2015-12-23 00:40:00 should be 2015-12-23T00:40:00.000 ; changing now.
Messed up time: 12473 M2.3 2015-01-02 00:10:00 should be 2016-01-02T00:11:00.000 ; changing now.
DONKI flare peak time 2016-07-23 05:16:00 has no match; dropping row.
DONKI flare peak time 2016-07-23 05:31:00 has no match; dropping row.
Messed up time: 12644 M5.3 2017-04-02 08:02:00 should be 2017-04-02T08:02:00.000 ; changing now.
Messed up time: 12644 M1.2 2017-04-03 01:05:00 should be 2017-04-03T01:05:00.000 ; changing now.
Messed up time: 12673 M7.3 2017-09-07 10:15:00 should be 2017-09-07T10:15:00.000 ; changing now.
There are 71 DONKI mistakes so far.
This is our final table of events that fall into the positive class:
events_list
flrID | instruments | beginTime | peakTime | endTime | classType | sourceLocation | activeRegionNum | linkedEvents | link | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 2011-02-15T01:44:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-02-15T01:44Z | 2011-02-15T01:56:00.000 | 2011-02-15T02:06Z | X2.2 | S20W10 | 11158.0 | [{'activityID': '2011-02-15T02:25:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
1 | 2011-02-24T07:23:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-02-24T07:23Z | 2011-02-24 07:35:00 | 2011-02-24T07:42Z | M3.5 | N14E87 | 11163.0 | [{'activityID': '2011-02-24T08:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
2 | 2011-03-07T13:44:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-03-07T13:44Z | 2011-03-07 14:30:00 | 2011-03-07T15:08Z | M2.0 | N11E21 | 11166.0 | [{'activityID': '2011-03-07T14:40:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
3 | 2011-03-07T19:43:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-03-07T19:43Z | 2011-03-07 20:12:00 | 2011-03-07T21:40Z | M3.7 | N30W48 | 11164.0 | [{'activityID': '2011-03-07T20:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
4 | 2011-03-08T03:37:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-03-08T03:37Z | 2011-03-08 03:58:00 | 2011-03-08T04:20Z | M1.5 | S21E72 | 11171.0 | [{'activityID': '2011-03-08T05:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
5 | 2011-06-07T06:16:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-06-07T06:16Z | 2011-06-07 06:41:00 | 2011-06-07T06:59Z | M2.5 | S22W53 | 11226.0 | [{'activityID': '2011-06-07T06:50:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
6 | 2011-08-03T13:17:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-08-03T13:17Z | 2011-08-03 13:48:00 | 2011-08-03T14:10Z | M6.0 | N17W29 | 11261.0 | [{'activityID': '2011-08-03T13:55:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
7 | 2011-08-04T03:41:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-08-04T03:41Z | 2011-08-04 03:57:00 | 2011-08-04T04:04Z | M9.3 | N15W39 | 11261.0 | [{'activityID': '2011-08-04T04:10:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
8 | 2011-09-07T22:32:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-09-07T22:32Z | 2011-09-07 22:38:00 | 2011-09-07T22:44Z | X1.8 | N15W31 | 11283.0 | [{'activityID': '2011-09-07T23:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
9 | 2011-09-22T10:29:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2011-09-22T10:29Z | 2011-09-22T11:01:00.000 | 2011-09-22T11:44Z | X1.4 | N09E89 | 11302.0 | [{'activityID': '2011-09-22T11:24:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
10 | 2012-01-23T03:38:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-01-23T03:38Z | 2012-01-23 03:59:00 | 2012-01-23T04:34Z | M8.7 | N28W20 | 11402.0 | [{'activityID': '2012-01-23T04:00:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
11 | 2012-01-27T17:37:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-01-27T17:37Z | 2012-01-27 18:37:00 | 2012-01-27T18:56Z | X1.8 | N31W90 | 11402.0 | [{'activityID': '2012-01-27T16:39:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
12 | 2012-03-05T03:30:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-03-05T03:30Z | 2012-03-05T04:09:00.000 | 2012-03-05T04:43Z | X1.1 | N19W58 | 11429.0 | [{'activityID': '2012-03-05T04:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
13 | 2012-03-07T00:00:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-03-07T00:02Z | 2012-03-07T00:24:00.000 | 2012-03-07T00:40Z | X5.4 | N18E31 | 11429.0 | [{'activityID': '2012-03-07T00:36:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
14 | 2012-03-07T01:05:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-03-07T01:05Z | 2012-03-07T01:14:00.000 | 2012-03-07T01:23Z | X1.3 | N17E27 | 11430.0 | [{'activityID': '2012-03-07T00:36:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
15 | 2012-03-09T03:22:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-03-09T03:22Z | 2012-03-09 03:53:00 | 2012-03-09T04:18Z | M6.3 | N17E02 | 11429.0 | [{'activityID': '2012-03-09T04:25:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
16 | 2012-03-10T17:32:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-03-10T17:32Z | 2012-03-10 17:44:00 | 2012-03-10T18:30Z | M8.4 | N18W22 | 11429.0 | [{'activityID': '2012-03-10T18:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
17 | 2012-03-13T16:21:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-03-13T16:21Z | 2012-03-13 17:41:00 | 2012-03-13T18:25Z | M7.9 | N18W59 | 11429.0 | [{'activityID': '2012-03-13T17:52:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
18 | 2012-05-17T00:30:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-05-17T00:30Z | 2012-05-17T01:47:00.000 | 2012-05-17T02:14Z | M5.1 | N06W90 | 11476.0 | [{'activityID': '2012-05-17T01:48:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
19 | 2012-06-13T11:29:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-06-13T11:29Z | 2012-06-13 13:17:00 | 2012-06-13T14:31Z | M1.2 | S18E21 | 11504.0 | [{'activityID': '2012-06-13T14:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
20 | 2012-06-14T12:52:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-06-14T12:52Z | 2012-06-14 14:35:00 | 2012-06-14T15:56Z | M1.9 | S17W00 | 11504.0 | [{'activityID': '2012-06-14T14:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
21 | 2012-07-06T23:01:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-07-06T23:01Z | 2012-07-06 23:08:00 | 2012-07-06T23:14Z | X1.1 | S17W50 | 11515.0 | [{'activityID': '2012-07-06T23:12:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
22 | 2012-07-12T15:37:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-07-12T15:37Z | 2012-07-12 16:49:00 | 2012-07-12T17:30Z | X1.4 | S14W02 | 11520.0 | [{'activityID': '2012-07-12T16:54:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
23 | 2012-07-17T12:03:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-07-17T12:03Z | 2012-07-17 17:15:00 | 2012-07-17T19:04Z | M1.8 | S15W88 | 11520.0 | [{'activityID': '2012-07-17T14:24:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
24 | 2012-07-19T04:17:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-07-19T04:17Z | 2012-07-19 05:58:00 | 2012-07-19T06:56Z | M7.7 | S17W91 | 11520.0 | [{'activityID': '2012-07-19T05:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
25 | 2012-07-28T20:44:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-07-28T20:44Z | 2012-07-28 20:56:00 | 2012-07-28T21:04Z | M6.2 | S20E49 | 11532.0 | [{'activityID': '2012-07-28T21:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
26 | 2012-11-08T02:09:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-11-08T02:09Z | 2012-11-08 02:23:00 | 2012-11-08T04:40Z | M1.7 | S10E70 | 11611.0 | [{'activityID': '2012-11-08T11:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
27 | 2012-11-21T15:10:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2012-11-21T15:10Z | 2012-11-21 15:30:00 | 2012-11-21T15:38Z | M3.5 | N08W00 | 11618.0 | [{'activityID': '2012-11-21T16:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
28 | 2013-04-11T06:55:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-04-11T06:55Z | 2013-04-11T07:16:00.000 | 2013-04-11T07:29Z | M6.5 | N07E13 | 11719.0 | [{'activityID': '2013-04-11T07:36:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
29 | 2013-05-13T01:53:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-05-13T01:53Z | 2013-05-13 02:17:00 | 2013-05-13T02:32Z | X1.6 | N10E89 | 11748.0 | [{'activityID': '2013-05-13T02:54:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
30 | 2013-05-13T15:40:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-05-13T15:40Z | 2013-05-13 16:05:00 | 2013-05-13T16:16Z | X2.8 | N10E89 | 11748.0 | [{'activityID': '2013-05-13T16:18:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
31 | 2013-05-14T01:00:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-05-14T01:00Z | 2013-05-14T01:11:00.000 | 2013-05-14T01:20Z | X3.2 | N10E89 | 11748.0 | [{'activityID': '2013-05-14T01:30:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
32 | 2013-05-15T01:25:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-05-15T01:25Z | 2013-05-15T01:48:00.000 | 2013-05-15T01:58Z | X1.2 | N11E63 | 11748.0 | [{'activityID': '2013-05-15T02:18:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
33 | 2013-05-22T12:30:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-05-22T12:30Z | 2013-05-22T13:32:00.000 | 2013-05-22T17:10Z | M5.0 | N13W75 | 11745.0 | [{'activityID': '2013-05-22T13:24:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
34 | 2013-10-22T21:15:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-22T21:15Z | 2013-10-22 21:20:00 | 2013-10-22T21:32Z | M4.3 | N05E03 | 11875.0 | [{'activityID': '2013-10-22T22:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
35 | 2013-10-24T00:22:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-24T00:22Z | 2013-10-24T00:30:00.000 | 2013-10-24T00:35Z | M9.3 | S13E09 | 11877.0 | [{'activityID': '2013-10-24T01:48:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
36 | 2013-10-25T07:53:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-25T07:53Z | 2013-10-25T08:01:00.000 | 2013-10-25T08:09Z | X1.7 | S08E73 | 11882.0 | [{'activityID': '2013-10-25T08:24:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
37 | 2013-10-25T14:52:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-25T14:52Z | 2013-10-25 15:03:00 | 2013-10-25T15:12Z | X2.1 | S08E65 | 11882.0 | [{'activityID': '2013-10-25T15:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
38 | 2013-10-26T19:24:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-26T19:24Z | 2013-10-26 19:27:00 | 2013-10-26T19:30Z | M3.1 | S09E77 | 11884.0 | [{'activityID': '2013-10-26T20:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
39 | 2013-10-28T01:41:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-28T01:41Z | 2013-10-28 02:03:00 | 2013-10-28T02:12Z | X1.0 | N07W634 | 11875.0 | [{'activityID': '2013-10-28T02:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
40 | 2013-10-28T04:32:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-28T04:32Z | 2013-10-28 04:41:00 | 2013-10-28T04:46Z | M5.1 | N07W65 | 11875.0 | [{'activityID': '2013-10-28T04:48:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
41 | 2013-10-29T21:48:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-10-29T21:48Z | 2013-10-29 21:54:00 | 2013-10-29T22:01Z | X2.3 | N07W89 | 11875.0 | [{'activityID': '2013-10-29T22:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
42 | 2013-11-08T04:20:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-11-08T04:20Z | 2013-11-08 04:26:00 | 2013-11-08T04:29Z | X1.1 | S10E11 | 11890.0 | [{'activityID': '2013-11-08T06:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
43 | 2013-11-10T05:08:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-11-10T05:08Z | 2013-11-10 05:14:00 | 2013-11-10T05:18Z | X1.1 | S11W16 | 11890.0 | [{'activityID': '2013-11-10T05:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
44 | 2013-11-19T10:14:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2013-11-19T10:14Z | 2013-11-19 10:26:00 | 2013-11-19T10:34Z | X1.0 | S13W69 | 11893.0 | [{'activityID': '2013-11-19T10:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
45 | 2014-01-04T18:47:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-01-04T18:47Z | 2014-01-04 19:46:00 | 2014-01-04T20:23Z | M4.0 | S11E33 | 11943.0 | [{'activityID': '2014-01-04T21:25:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
46 | 2014-01-04T22:12:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-01-04T22:12Z | 2014-01-04 22:52:00 | 2014-01-04T23:22Z | M1.9 | S14W89 | 11936.0 | [{'activityID': '2014-01-05T00:54:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
47 | 2014-01-07T18:02:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-01-07T18:02Z | 2014-01-07 18:32:00 | 2014-01-07T18:58Z | X1.2 | S15W10 | 11944.0 | [{'activityID': '2014-01-07T18:24:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
48 | 2014-01-08T03:39:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-01-08T03:39Z | 2014-01-08 03:47:00 | 2014-01-08T03:54Z | M3.6 | N11W88 | 11947.0 | [{'activityID': '2014-01-08T04:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
49 | 2014-01-30T07:54:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-01-30T07:54Z | 2014-01-30 08:11:00 | 2014-01-30T08:41Z | M1.1 | S14E45 | 11967.0 | [{'activityID': '2014-01-30T08:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
50 | 2014-01-30T15:48:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-01-30T15:48Z | 2014-01-30 16:11:00 | 2014-01-30T16:28Z | M6.6 | S12E61 | 11967.0 | [{'activityID': '2014-01-30T16:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
51 | 2014-02-11T03:22:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-02-11T03:22Z | 2014-02-11 03:31:00 | 2014-02-11T03:39Z | M1.7 | S13E16 | 11974.0 | [{'activityID': '2014-02-11T05:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
52 | 2014-02-25T00:41:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-02-25T00:41Z | 2014-02-25 00:49:00 | 2014-02-25T01:03Z | X4.9 | S15E77 | 11990.0 | [{'activityID': '2014-02-25T01:25:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
53 | 2014-03-29T17:36:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-03-29T17:36Z | 2014-03-29 17:48:00 | 2014-03-29T17:54Z | X1.0 | N10W32 | 12017.0 | [{'activityID': '2014-03-29T18:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
54 | 2014-04-02T13:18:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-04-02T13:18Z | 2014-04-02 14:05:00 | 2014-04-02T14:28Z | M6.5 | N12E53 | 12027.0 | [{'activityID': '2014-04-02T13:55:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
55 | 2014-04-18T12:31:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-04-18T12:31Z | 2014-04-18 13:03:00 | 2014-04-18T13:20Z | M7.3 | S18W29 | 12036.0 | [{'activityID': '2014-04-18T13:09:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
56 | 2014-04-25T00:17:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-04-25T00:17Z | 2014-04-25T00:27:00.000 | 2014-04-25T00:38Z | X1.3 | S14W29 | 12035.0 | [{'activityID': '2014-04-25T00:48:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
57 | 2014-05-06T08:40:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-05-06T08:40Z | 2014-05-06 09:03:00 | 2014-05-06T09:24Z | M1.8 | S13W87 | 12051.0 | [{'activityID': '2014-05-06T09:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
58 | 2014-05-06T22:01:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-05-06T22:01Z | 2014-05-06 22:09:00 | 2014-05-06T22:20Z | M1.0 | S09W91 | 12051.0 | [{'activityID': '2014-05-06T22:17:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
59 | 2014-05-07T16:07:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-05-07T16:07Z | 2014-05-07 16:29:00 | 2014-05-07T13:03Z | M1.2 | S12W90 | 12055.0 | [{'activityID': '2014-05-07T16:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
60 | 2014-06-10T11:38:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-06-10T11:38Z | 2014-06-10T11:42:00.000 | 2014-06-10T11:47Z | X2.2 | S18E85 | 12087.0 | [{'activityID': '2014-06-10T12:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
61 | 2014-06-10T12:38:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-06-10T12:38Z | 2014-06-10 12:52:00 | 2014-06-10T13:07Z | X1.5 | S19E89 | 12087.0 | [{'activityID': '2014-06-10T13:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
62 | 2014-06-12T21:39:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-06-12T21:39Z | 2014-06-12 22:16:00 | 2014-06-12T22:52Z | M3.1 | S23W58 | 12085.0 | [{'activityID': '2014-06-12T22:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
63 | 2014-07-01T11:04:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-07-01T11:04Z | 2014-07-01 11:23:00 | 2014-07-01T11:50Z | M1.4 | N12E60 | 12106.0 | [{'activityID': '2014-07-01T12:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
64 | 2014-07-08T16:08:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-07-08T16:08Z | 2014-07-08 16:20:00 | 2014-07-08T16:30Z | M6.5 | N12E56 | 12113.0 | [{'activityID': '2014-07-08T16:54:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
65 | 2014-08-01T18:00:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-08-01T18:00Z | 2014-08-01T18:13:00.000 | 2014-08-01T18:48Z | M1.5 | S09E13 | 12127.0 | [{'activityID': '2014-08-01T18:54:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
66 | 2014-08-21T13:19:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-08-21T13:19Z | 2014-08-21 13:31:00 | 2014-08-21T13:31Z | M3.4 | N11E90 | 12149.0 | [{'activityID': '2014-08-21T14:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
67 | 2014-08-25T14:46:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-08-25T14:46Z | 2014-08-25T15:11:00.000 | 2014-08-25T15:11Z | M2.0 | N06W39 | 12146.0 | [{'activityID': '2014-08-25T15:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
68 | 2014-08-25T20:06:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-08-25T20:06Z | 2014-08-25 20:21:00 | 2014-08-25T20:29Z | M3.9 | N07W43 | 12146.0 | [{'activityID': '2014-08-25T21:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
69 | 2014-09-10T17:21:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-09-10T17:21Z | 2014-09-10 17:45:00 | 2014-09-10T18:20Z | X1.6 | N15E05 | 12158.0 | [{'activityID': '2014-09-10T18:18:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
70 | 2014-09-14T02:03:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-09-14T02:03Z | 2014-09-14 02:16:00 | 2014-09-14T02:36Z | M1.5 | S14W49 | 12157.0 | [{'activityID': '2014-09-14T03:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
71 | 2014-09-23T23:02:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-09-23T23:02Z | 2014-09-23T23:16:00.000 | 2014-09-23T23:28Z | M2.3 | S30W35 | 12172.0 | [{'activityID': '2014-09-24T00:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
72 | 2014-09-28T02:39:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-09-28T02:39Z | 2014-09-28 02:58:00 | 2014-09-28T03:19Z | M5.1 | S11W17 | 12173.0 | [{'activityID': '2014-09-28T03:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
73 | 2014-10-02T18:49:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-10-02T18:49Z | 2014-10-02 19:01:00 | 2014-10-02T19:14Z | M7.3 | S14W88 | 12173.0 | [{'activityID': '2014-10-02T19:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
74 | 2014-10-16T12:58:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-10-16T12:58Z | 2014-10-16 13:03:00 | 2014-10-16T13:05Z | M4.3 | S13E88 | 12192.0 | [{'activityID': '2014-10-16T13:26:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
75 | 2014-10-24T07:37:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-10-24T07:37Z | 2014-10-24T07:48:00.000 | 2014-10-24T07:53Z | M4.0 | S19W05 | 12192.0 | [{'activityID': '2014-10-24T08:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
76 | 2014-11-03T11:23:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-03T11:23Z | 2014-11-03 11:53:00 | 2014-11-03T12:17Z | M2.2 | N17E89 | 12205.0 | [{'activityID': '2014-11-03T12:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
77 | 2014-11-03T22:15:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-03T22:15Z | 2014-11-03 22:40:00 | 2014-11-03T22:53Z | M6.5 | N18E90 | 12205.0 | [{'activityID': '2014-11-03T23:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
78 | 2014-11-04T07:59:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-04T07:59Z | 2014-11-04T08:38:00.000 | 2014-11-04T08:51Z | M2.6 | N14E89 | 12205.0 | [{'activityID': '2014-11-04T09:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
79 | 2014-11-04T08:52:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-04T08:52Z | 2014-11-04 09:04:00 | 2014-11-04T09:13Z | M2.3 | N13E89 | 12205.0 | [{'activityID': '2014-11-04T09:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
80 | 2014-11-05T09:26:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-05T09:26Z | 2014-11-05 09:47:00 | 2014-11-05T09:55Z | M7.9 | N16E63 | 12205.0 | [{'activityID': '2014-11-05T10:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
81 | 2014-11-05T18:50:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-05T18:50Z | 2014-11-05 19:44:00 | 2014-11-05T20:15Z | M2.9 | N16E69 | 12205.0 | [{'activityID': '2014-11-05T20:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
82 | 2014-11-06T03:32:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-06T03:32Z | 2014-11-06 03:46:00 | 2014-11-06T04:02Z | M5.4 | N16E53 | 12205.0 | [{'activityID': '2014-11-06T04:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
83 | 2014-11-07T04:12:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-07T04:12Z | 2014-11-07 04:25:00 | 2014-11-07T04:38Z | M2.0 | N17E45 | 12205.0 | [{'activityID': '2014-11-07T04:17:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
84 | 2014-11-07T16:53:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-07T16:53Z | 2014-11-07 17:26:00 | 2014-11-07T17:34Z | X1.6 | N15E35 | 12205.0 | [{'activityID': '2014-11-07T18:08:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
85 | 2014-11-15T20:38:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-11-15T20:38Z | 2014-11-15 20:46:00 | 2014-11-15T20:50Z | M3.7 | S15E44 | 12209.0 | [{'activityID': '2014-11-15T21:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
86 | 2014-12-17T01:41:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-12-17T01:41Z | 2014-12-17T01:50:00.000 | 2014-12-17T01:57Z | M1.1 | S11E37 | 12241.0 | [{'activityID': '2014-12-17T02:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
87 | 2014-12-17T04:25:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-12-17T04:25Z | 2014-12-17T04:51:00.000 | 2014-12-17T05:20Z | M8.7 | S18E08 | 12242.0 | [{'activityID': '2014-12-17T05:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
88 | 2014-12-18T21:41:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-12-18T21:41Z | 2014-12-18 21:58:00 | 2014-12-18T22:25Z | M6.9 | S11E10 | 12241.0 | [{'activityID': '2014-12-19T00:27:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
89 | 2014-12-20T00:11:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2014-12-20T00:11Z | 2014-12-20T00:28:00.000 | 2014-12-20T00:55Z | X1.8 | S19W28 | 12242.0 | [{'activityID': '2014-12-20T01:25:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
90 | 2015-01-13T04:13:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-01-13T04:13Z | 2015-01-13 04:24:00 | 2015-01-13T04:38Z | M5.6 | N07W69 | 12257.0 | [{'activityID': '2015-01-13T05:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
91 | 2015-02-09T22:59:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-02-09T22:59Z | 2015-02-09 23:35:00 | 2015-02-10T00:12Z | M2.4 | N15E52 | 12280.0 | [{'activityID': '2015-02-09T23:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
92 | 2015-03-06T04:14:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-03-06T04:14Z | 2015-03-06T04:57:00.000 | 2015-03-06T05:27Z | M3.0 | S20E90 | 12297.0 | [{'activityID': '2015-03-06T04:49:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
93 | 2015-03-06T06:55:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-03-06T06:55Z | 2015-03-06 08:15:00 | 2015-03-06T08:28Z | M1.5 | S20E90 | 12297.0 | [{'activityID': '2015-03-06T07:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
94 | 2015-03-07T21:45:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-03-07T21:45Z | 2015-03-07 22:22:00 | 2015-03-07T22:58Z | M9.2 | S20E78 | 12297.0 | [{'activityID': '2015-03-07T22:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
95 | 2015-03-09T23:29:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-03-09T23:29Z | 2015-03-09 23:53:00 | 2015-03-10T00:12Z | M5.8 | S17E39 | 12297.0 | [{'activityID': '2015-03-10T00:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
96 | 2015-03-10T03:19:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-03-10T03:19Z | 2015-03-10T03:24:00.000 | 2015-03-10T03:28Z | M5.1 | S16E38 | 12297.0 | [{'activityID': '2015-03-10T03:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
97 | 2015-03-11T16:11:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-03-11T16:11Z | 2015-03-11 16:22:00 | 2015-03-11T16:29Z | X2.2 | S16E26 | 12297.0 | [{'activityID': '2015-03-11T18:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
98 | 2015-03-17T22:49:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-03-17T22:49Z | 2015-03-17 23:34:00 | 2015-03-17T23:48Z | M1.0 | S17W64 | 12297.0 | [{'activityID': '2015-03-18T00:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
99 | 2015-04-12T08:51:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-04-12T08:51Z | 2015-04-12 09:50:00 | 2015-04-12T10:44Z | M1.1 | N11E78 | 12321.0 | [{'activityID': '2015-04-12T10:00:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
100 | 2015-04-23T09:18:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-04-23T09:18Z | 2015-04-12T09:50:00.000 | 2015-04-23T10:07Z | M1.1 | N07W80 | 12321.0 | [{'activityID': '2015-04-23T09:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
101 | 2015-05-05T22:05:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-05-05T22:05Z | 2015-05-05 22:11:00 | 2015-05-05T22:15Z | X2.7 | N15E79 | 12339.0 | [{'activityID': '2015-05-05T22:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
102 | 2015-06-18T16:33:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-06-18T16:33Z | 2015-06-18 17:36:00 | 2015-06-18T18:25Z | M3.0 | N15E45 | 12371.0 | [{'activityID': '2015-06-18T17:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
103 | 2015-06-21T01:02:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-06-21T01:02Z | 2015-06-21 01:42:00 | 2015-06-21T02:00Z | M2.0 | N13E12 | 12371.0 | [{'activityID': '2015-06-21T02:48:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
104 | 2015-06-21T02:06:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-06-21T02:06Z | 2015-06-21T02:36:00.000 | 2015-06-21T03:02Z | M2.6 | N13E12 | 12371.0 | [{'activityID': '2015-06-21T02:48:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
105 | 2015-06-22T17:39:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-06-22T17:39Z | 2015-06-22 18:23:00 | 2015-06-22T18:51Z | M6.5 | N13W05 | 12371.0 | [{'activityID': '2015-06-22T18:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
106 | 2015-06-25T08:02:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-06-25T08:02Z | 2015-06-25 08:16:00 | 2015-06-25T09:05Z | M7.9 | N13W40 | 12371.0 | [{'activityID': '2015-06-25T08:36:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
107 | 2015-08-21T09:34:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-08-21T09:34Z | 2015-08-21T09:48:00.000 | 2015-08-21T10:07Z | M1.4 | S17E26 | 12403.0 | [{'activityID': '2015-08-21T10:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
108 | 2015-08-22T06:39:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-08-22T06:39Z | 2015-08-21T02:18:00.000 | 2015-08-22T06:59Z | M1.2 | S15E13 | 12403.0 | [{'activityID': '2015-08-22T07:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
109 | 2015-09-20T17:32:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-09-20T17:32Z | 2015-09-20 18:03:00 | 2015-09-20T18:29Z | M2.1 | S22W50 | 12415.0 | [{'activityID': '2015-09-20T18:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
110 | 2015-09-28T03:45:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-09-28T03:45Z | 2015-09-28T03:55:00.000 | 2015-09-28T03:59Z | M3.6 | S08W71 | 12423.0 | [{'activityID': '2015-09-28T05:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
111 | 2015-11-04T03:20:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-11-04T03:20Z | 2015-11-04T03:26:00.000 | 2015-11-04T03:29Z | M1.9 | N14W65 | 12445.0 | [{'activityID': '2015-11-04T03:48:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
112 | 2015-11-04T13:30:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-11-04T13:30Z | 2015-11-04 13:52:00 | 2015-11-04T14:13Z | M3.7 | N06W04 | 12443.0 | [{'activityID': '2015-11-04T14:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
113 | 2015-12-21T00:52:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-12-21T00:52Z | 2015-12-21T01:03:00.000 | 2015-12-21T01:11Z | M2.8 | N01E81 | 12472.0 | [{'activityID': '2015-12-21T01:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
114 | 2015-12-21T10:09:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-12-21T10:09Z | 2015-12-21T10:19:00.000 | 2015-12-21T10:32Z | M1.1 | N01E81 | 12472.0 | [{'activityID': '2015-12-21T10:48:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
115 | 2015-12-23T00:23:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-12-23T00:23Z | 2015-12-23T00:40:00.000 | 2015-12-23T00:52Z | M4.7 | S21E65 | 12473.0 | [{'activityID': '2015-12-23T01:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
116 | 2015-12-28T11:20:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2015-12-28T11:20Z | 2015-12-28 12:45:00 | 2015-12-28T14:09Z | M1.8 | S22W12 | 12473.0 | [{'activityID': '2015-12-28T12:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
117 | 2016-01-01T23:00:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2016-01-01T23:00Z | 2016-01-02T00:11:00.000 | None | M2.3 | S21W73 | 12473.0 | [{'activityID': '2016-01-01T23:12:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
118 | 2016-04-18T00:14:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2016-04-18T00:14Z | 2016-04-18 00:29:00 | 2016-04-18T00:39Z | M6.7 | N10W51 | 12529.0 | [{'activityID': '2016-04-18T00:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
119 | 2017-04-01T21:35:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-04-01T21:35Z | 2017-04-01 21:48:00 | 2017-04-01T22:05Z | M4.4 | S13W54 | 12644.0 | [{'activityID': '2017-04-01T22:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
120 | 2017-04-02T07:48:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-04-02T07:48Z | 2017-04-02T08:02:00.000 | 2017-04-02T08:13Z | M5.3 | N12W56 | 12644.0 | [{'activityID': '2017-04-02T09:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
121 | 2017-04-02T18:18:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-04-02T18:18Z | 2017-04-02 18:38:00 | 2017-04-02T19:28Z | M2.1 | N12W66 | 12644.0 | [{'activityID': '2017-04-02T20:09:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
122 | 2017-04-03T00:54:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-04-03T00:54Z | 2017-04-03T01:05:00.000 | 2017-04-03T01:12Z | M1.2 | N12W66 | 12644.0 | [{'activityID': '2017-04-03T01:48:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
123 | 2017-04-03T14:21:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-04-03T14:21Z | 2017-04-03 14:29:00 | 2017-04-03T14:34Z | M5.8 | N16W78 | 12644.0 | [{'activityID': '2017-04-03T15:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
124 | 2017-07-14T01:07:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-07-14T01:07Z | 2017-07-14 02:09:00 | 2017-07-14T03:24Z | M2.4 | S09W33 | 12665.0 | [{'activityID': '2017-07-14T01:36:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
125 | 2017-09-04T15:11:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-04T15:11Z | 2017-09-04 15:30:00 | 2017-09-04T15:33Z | M1.5 | S10W08 | 12673.0 | [{'activityID': '2017-09-04T19:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
126 | 2017-09-04T18:05:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-04T18:05Z | 2017-09-04 18:22:00 | 2019-09-04T18:31Z | M1.0 | S10W12 | 12673.0 | [{'activityID': '2017-09-04T19:39:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
127 | 2017-09-04T20:15:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-04T20:15Z | 2017-09-04 20:33:00 | 2017-09-04T20:37Z | M5.5 | S10W14 | 12673.0 | [{'activityID': '2017-09-04T20:36:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
128 | 2017-09-06T11:53:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-06T11:53Z | 2017-09-06 12:02:00 | 2017-09-06T12:10Z | X9.3 | S07W33 | 12673.0 | [{'activityID': '2017-09-06T12:24:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
129 | 2017-09-07T10:11:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-07T10:11Z | 2017-09-07T10:15:00.000 | 2017-09-07T10:18Z | M7.3 | S08W47 | 12673.0 | [{'activityID': '2017-09-07T10:48:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
130 | 2017-09-07T14:20:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-07T14:20Z | 2017-09-07 14:36:00 | 2017-09-07T14:55Z | X1.3 | S09W53 | 12673.0 | [{'activityID': '2017-09-07T15:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
131 | 2017-09-07T23:51:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-07T23:51Z | 2017-09-07 23:59:00 | 2017-09-08T00:14Z | M3.9 | S15W50 | 12673.0 | [{'activityID': '2017-09-07T23:36:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
132 | 2017-09-08T03:39:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-08T03:39Z | 2017-09-08 03:43:00 | 2017-09-08T03:46Z | M1.2 | S07W55 | 12673.0 | [{'activityID': '2017-09-08T04:17:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
133 | 2017-09-08T07:40:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-08T07:40Z | 2017-09-08 07:49:00 | 2017-09-08T07:58Z | M8.1 | S10W58 | 12673.0 | [{'activityID': '2017-09-08T07:24:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
134 | 2017-09-10T15:35:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-09-10T15:35Z | 2017-09-10 16:06:00 | 2017-09-10T16:31Z | X8.2 | S08W88 | 12673.0 | [{'activityID': '2017-09-10T16:09:00-CME-001'}... | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
135 | 2017-10-20T23:10:00-FLR-001 | [{'id': 11, 'displayName': 'GOES15: SEM/XRS 1.... | 2017-10-20T23:10Z | 2017-10-20 23:28:00 | 2017-10-21T23:37Z | M1.1 | S10E90 | 12685.0 | [{'activityID': '2017-10-21T00:12:00-CME-001'}] | https://kauai.ccmc.gsfc.nasa.gov/DONKI/view/FL... |
Now let’s query the JSOC database to see if there are active region parameters at the time of the flare. First read the following file to map NOAA active region numbers to HARPNUMs (a HARP, or an HMI Active Region Patch, is the preferred numbering system for the HMI active regions as they appear in the magnetic field data before NOAA observes them in white light):
answer = pd.read_csv(
'http://jsoc.stanford.edu/doc/data/hmi/harpnum_to_noaa/all_harps_with_noaa_ars.txt', sep=' ')
Now, let’s determine at which time we’d like to predict CMEs. In general, many people try to predict a CME either 24 or 48 hours before it happens. We can report both in this study by setting a variable called timedelayvariable
:
timedelayvariable = 24
Now, we’ll convert subtract timedelayvariable
from the GOES Peak Time and re-format the datetime object into a string that JSOC can understand:
t_rec = [(events_list['peakTime'].iloc[i] - timedelta(hours=timedelayvariable)
).strftime('%Y.%m.%d_%H:%M_TAI') for i in range(events_list.shape[0])]
Now we can grab the SDO data from the JSOC database by executing the JSON queries. We are selecting data that satisfies several criteria: The data has to be [1] disambiguated with a version of the disambiguation module greater than 1.1, [2] taken while the orbital velocity of the spacecraft is less than 3500 m/s, [3] of a high quality, and [4] within 70 degrees of central meridian. If the data pass all these tests, they are stuffed into one of two lists: one for the positive class (called CME_data) and one for the negative class (called no_CME_data).
def get_the_jsoc_data(event_count, t_rec):
"""
Parameters
----------
event_count: number of events
int
t_rec: list of times, one associated with each event in event_count
list of strings in JSOC format ('%Y.%m.%d_%H:%M_TAI')
"""
catalog_data = []
classification = []
for i in range(event_count):
print("=====", i, "=====")
# next match NOAA_ARS to HARPNUM
idx = answer[answer['NOAA_ARS'].str.contains(
str(int(listofactiveregions[i])))]
# if there's no HARPNUM, quit
if (idx.empty == True):
print('skip: there are no matching HARPNUMs for',
str(int(listofactiveregions[i])))
continue
# construct jsoc_info queries and query jsoc database; we are querying for 25 keywords
url = "http://jsoc.stanford.edu/cgi-bin/ajax/jsoc_info?ds=hmi.sharp_720s["+str(
idx.HARPNUM.values[0])+"]["+t_rec[i]+"][? (CODEVER7 !~ '1.1 ') and (abs(OBS_VR)< 3500) and (QUALITY<65536) ?]&op=rs_list&key=USFLUX,MEANGBT,MEANJZH,MEANPOT,SHRGT45,TOTUSJH,MEANGBH,MEANALP,MEANGAM,MEANGBZ,MEANJZD,TOTUSJZ,SAVNCPP,TOTPOT,MEANSHR,AREA_ACR,R_VALUE,ABSNJZH"
response = requests.get(url)
# if there's no response at this time, quit
if response.status_code != 200:
print('skip: cannot successfully get an http response')
continue
# read the JSON output
data = response.json()
# if there are no data at this time, quit
if data['count'] == 0:
print('skip: there are no data for HARPNUM',
idx.HARPNUM.values[0], 'at time', t_rec[i])
continue
# check to see if the active region is too close to the limb
# we can compute the latitude of an active region in stonyhurst coordinates as follows:
# latitude_stonyhurst = CRVAL1 - CRLN_OBS
# for this we have to query the CEA series (but above we queried the other series as the CEA series does not have CODEVER5 in it)
url = "http://jsoc.stanford.edu/cgi-bin/ajax/jsoc_info?ds=hmi.sharp_cea_720s["+str(
idx.HARPNUM.values[0])+"]["+t_rec[i]+"][? (abs(OBS_VR)< 3500) and (QUALITY<65536) ?]&op=rs_list&key=CRVAL1,CRLN_OBS"
response = requests.get(url)
# if there's no response at this time, quit
if response.status_code != 200:
print('skip: failed to find CEA JSOC data for HARPNUM',
idx.HARPNUM.values[0], 'at time', t_rec[i])
continue
# read the JSON output
latitude_information = response.json()
# if there are no data at this time, quit
if latitude_information['count'] == 0:
print('skip: there are no data for HARPNUM',
idx.HARPNUM.values[0], 'at time', t_rec[i])
continue
CRVAL1 = float(latitude_information['keywords'][0]['values'][0])
CRLN_OBS = float(latitude_information['keywords'][1]['values'][0])
if (np.absolute(CRVAL1 - CRLN_OBS) > 70.0):
print('skip: latitude is out of range for HARPNUM',
idx.HARPNUM.values[0], 'at time', t_rec[i])
continue
if ('MISSING' in str(data['keywords'])):
print('skip: there are some missing keywords for HARPNUM',
idx.HARPNUM.values[0], 'at time', t_rec[i])
continue
print('accept NOAA Active Region number', str(int(
listofactiveregions[i])), 'and HARPNUM', idx.HARPNUM.values[0], 'at time', t_rec[i])
individual_flare_data = []
for j in range(18):
individual_flare_data.append(
float(data['keywords'][j]['values'][0]))
catalog_data.append(list(individual_flare_data))
single_class_instance = [idx.HARPNUM.values[0], str(
int(listofactiveregions[i])), listofgoesclasses[i], t_rec[i]]
classification.append(single_class_instance)
return catalog_data, classification
Now we prepare the data to be fed into the function:
listofactiveregions = list(events_list['activeRegionNum'].values.flatten())
listofgoesclasses = list(events_list['classType'].values.flatten())
And call the function:
positive_result = get_the_jsoc_data(events_list.shape[0], t_rec)
===== 0 =====
accept NOAA Active Region number 11158 and HARPNUM 377 at time 2011.02.14_01:56_TAI
===== 1 =====
skip: there are no data for HARPNUM 392 at time 2011.02.23_07:35_TAI
===== 2 =====
accept NOAA Active Region number 11166 and HARPNUM 401 at time 2011.03.06_14:30_TAI
===== 3 =====
accept NOAA Active Region number 11164 and HARPNUM 393 at time 2011.03.06_20:12_TAI
===== 4 =====
skip: there are no data for HARPNUM 415 at time 2011.03.07_03:58_TAI
===== 5 =====
accept NOAA Active Region number 11226 and HARPNUM 637 at time 2011.06.06_06:41_TAI
===== 6 =====
accept NOAA Active Region number 11261 and HARPNUM 750 at time 2011.08.02_13:48_TAI
===== 7 =====
accept NOAA Active Region number 11261 and HARPNUM 750 at time 2011.08.03_03:57_TAI
===== 8 =====
accept NOAA Active Region number 11283 and HARPNUM 833 at time 2011.09.06_22:38_TAI
===== 9 =====
skip: there are no data for HARPNUM 892 at time 2011.09.21_11:01_TAI
===== 10 =====
skip: there are some missing keywords for HARPNUM 1321 at time 2012.01.22_03:59_TAI
===== 11 =====
skip: latitude is out of range for HARPNUM 1321 at time 2012.01.26_18:37_TAI
===== 12 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.04_04:09_TAI
===== 13 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.06_00:24_TAI
===== 14 =====
accept NOAA Active Region number 11430 and HARPNUM 1449 at time 2012.03.06_01:14_TAI
===== 15 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.08_03:53_TAI
===== 16 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.09_17:44_TAI
===== 17 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.12_17:41_TAI
===== 18 =====
accept NOAA Active Region number 11476 and HARPNUM 1638 at time 2012.05.16_01:47_TAI
===== 19 =====
accept NOAA Active Region number 11504 and HARPNUM 1750 at time 2012.06.12_13:17_TAI
===== 20 =====
skip: there are no data for HARPNUM 1750 at time 2012.06.13_14:35_TAI
===== 21 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.05_23:08_TAI
===== 22 =====
accept NOAA Active Region number 11520 and HARPNUM 1834 at time 2012.07.11_16:49_TAI
===== 23 =====
accept NOAA Active Region number 11520 and HARPNUM 1834 at time 2012.07.16_17:15_TAI
===== 24 =====
skip: there are no data for HARPNUM 1834 at time 2012.07.18_05:58_TAI
===== 25 =====
accept NOAA Active Region number 11532 and HARPNUM 1879 at time 2012.07.27_20:56_TAI
===== 26 =====
skip: there are no data for HARPNUM 2186 at time 2012.11.07_02:23_TAI
===== 27 =====
accept NOAA Active Region number 11618 and HARPNUM 2220 at time 2012.11.20_15:30_TAI
===== 28 =====
accept NOAA Active Region number 11719 and HARPNUM 2635 at time 2013.04.10_07:16_TAI
===== 29 =====
skip: there are no data for HARPNUM 2748 at time 2013.05.12_02:17_TAI
===== 30 =====
skip: there are no data for HARPNUM 2748 at time 2013.05.12_16:05_TAI
===== 31 =====
skip: there are no data for HARPNUM 2748 at time 2013.05.13_01:11_TAI
===== 32 =====
skip: latitude is out of range for HARPNUM 2748 at time 2013.05.14_01:48_TAI
===== 33 =====
accept NOAA Active Region number 11745 and HARPNUM 2739 at time 2013.05.21_13:32_TAI
===== 34 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.21_21:20_TAI
===== 35 =====
accept NOAA Active Region number 11877 and HARPNUM 3295 at time 2013.10.23_00:30_TAI
===== 36 =====
skip: latitude is out of range for HARPNUM 3311 at time 2013.10.24_08:01_TAI
===== 37 =====
skip: there are no data for HARPNUM 3311 at time 2013.10.24_15:03_TAI
===== 38 =====
skip: there are no data for HARPNUM 3321 at time 2013.10.25_19:27_TAI
===== 39 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.27_02:03_TAI
===== 40 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.27_04:41_TAI
===== 41 =====
skip: latitude is out of range for HARPNUM 3291 at time 2013.10.28_21:54_TAI
===== 42 =====
accept NOAA Active Region number 11890 and HARPNUM 3341 at time 2013.11.07_04:26_TAI
===== 43 =====
accept NOAA Active Region number 11890 and HARPNUM 3341 at time 2013.11.09_05:14_TAI
===== 44 =====
skip: there are some missing keywords for HARPNUM 3364 at time 2013.11.18_10:26_TAI
===== 45 =====
accept NOAA Active Region number 11943 and HARPNUM 3563 at time 2014.01.03_19:46_TAI
===== 46 =====
skip: latitude is out of range for HARPNUM 3535 at time 2014.01.03_22:52_TAI
===== 47 =====
accept NOAA Active Region number 11944 and HARPNUM 3563 at time 2014.01.06_18:32_TAI
===== 48 =====
skip: latitude is out of range for HARPNUM 3587 at time 2014.01.07_03:47_TAI
===== 49 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.29_08:11_TAI
===== 50 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.29_16:11_TAI
===== 51 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.10_03:31_TAI
===== 52 =====
skip: latitude is out of range for HARPNUM 3793 at time 2014.02.24_00:49_TAI
===== 53 =====
accept NOAA Active Region number 12017 and HARPNUM 3894 at time 2014.03.28_17:48_TAI
===== 54 =====
accept NOAA Active Region number 12027 and HARPNUM 3941 at time 2014.04.01_14:05_TAI
===== 55 =====
accept NOAA Active Region number 12036 and HARPNUM 3999 at time 2014.04.17_13:03_TAI
===== 56 =====
accept NOAA Active Region number 12035 and HARPNUM 4000 at time 2014.04.24_00:27_TAI
===== 57 =====
accept NOAA Active Region number 12051 and HARPNUM 4071 at time 2014.05.05_09:03_TAI
===== 58 =====
skip: latitude is out of range for HARPNUM 4071 at time 2014.05.05_22:09_TAI
===== 59 =====
skip: latitude is out of range for HARPNUM 4097 at time 2014.05.06_16:29_TAI
===== 60 =====
skip: there are no data for HARPNUM 4225 at time 2014.06.09_11:42_TAI
===== 61 =====
skip: there are no data for HARPNUM 4225 at time 2014.06.09_12:52_TAI
===== 62 =====
accept NOAA Active Region number 12085 and HARPNUM 4197 at time 2014.06.11_22:16_TAI
===== 63 =====
accept NOAA Active Region number 12106 and HARPNUM 4294 at time 2014.06.30_11:23_TAI
===== 64 =====
skip: latitude is out of range for HARPNUM 4344 at time 2014.07.07_16:20_TAI
===== 65 =====
skip: there are no data for HARPNUM 4396 at time 2014.07.31_18:13_TAI
===== 66 =====
skip: there are no matching HARPNUMs for 12149
===== 67 =====
skip: there are no matching HARPNUMs for 12146
===== 68 =====
skip: there are no matching HARPNUMs for 12146
===== 69 =====
skip: there are no matching HARPNUMs for 12158
===== 70 =====
skip: there are no matching HARPNUMs for 12157
===== 71 =====
skip: there are no matching HARPNUMs for 12172
===== 72 =====
skip: there are no matching HARPNUMs for 12173
===== 73 =====
skip: there are no matching HARPNUMs for 12173
===== 74 =====
skip: there are no data for HARPNUM 4698 at time 2014.10.15_13:03_TAI
===== 75 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.23_07:48_TAI
===== 76 =====
skip: there are no data for HARPNUM 4781 at time 2014.11.02_11:53_TAI
===== 77 =====
skip: there are no data for HARPNUM 4781 at time 2014.11.02_22:40_TAI
===== 78 =====
skip: there are no data for HARPNUM 4781 at time 2014.11.03_08:38_TAI
===== 79 =====
skip: there are no data for HARPNUM 4781 at time 2014.11.03_09:04_TAI
===== 80 =====
skip: latitude is out of range for HARPNUM 4781 at time 2014.11.04_09:47_TAI
===== 81 =====
skip: latitude is out of range for HARPNUM 4781 at time 2014.11.04_19:44_TAI
===== 82 =====
skip: latitude is out of range for HARPNUM 4781 at time 2014.11.05_03:46_TAI
===== 83 =====
accept NOAA Active Region number 12205 and HARPNUM 4781 at time 2014.11.06_04:25_TAI
===== 84 =====
accept NOAA Active Region number 12205 and HARPNUM 4781 at time 2014.11.06_17:26_TAI
===== 85 =====
accept NOAA Active Region number 12209 and HARPNUM 4817 at time 2014.11.14_20:46_TAI
===== 86 =====
accept NOAA Active Region number 12241 and HARPNUM 4941 at time 2014.12.16_01:50_TAI
===== 87 =====
accept NOAA Active Region number 12242 and HARPNUM 4920 at time 2014.12.16_04:51_TAI
===== 88 =====
skip: there are no data for HARPNUM 4941 at time 2014.12.17_21:58_TAI
===== 89 =====
accept NOAA Active Region number 12242 and HARPNUM 4920 at time 2014.12.19_00:28_TAI
===== 90 =====
accept NOAA Active Region number 12257 and HARPNUM 5026 at time 2015.01.12_04:24_TAI
===== 91 =====
accept NOAA Active Region number 12280 and HARPNUM 5144 at time 2015.02.08_23:35_TAI
===== 92 =====
skip: there are no data for HARPNUM 5298 at time 2015.03.05_04:57_TAI
===== 93 =====
skip: there are no data for HARPNUM 5298 at time 2015.03.05_08:15_TAI
===== 94 =====
skip: latitude is out of range for HARPNUM 5298 at time 2015.03.06_22:22_TAI
===== 95 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.08_23:53_TAI
===== 96 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.09_03:24_TAI
===== 97 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.10_16:22_TAI
===== 98 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.16_23:34_TAI
===== 99 =====
skip: latitude is out of range for HARPNUM 5447 at time 2015.04.11_09:50_TAI
===== 100 =====
skip: latitude is out of range for HARPNUM 5447 at time 2015.04.11_09:50_TAI
===== 101 =====
skip: latitude is out of range for HARPNUM 5541 at time 2015.05.04_22:11_TAI
===== 102 =====
accept NOAA Active Region number 12371 and HARPNUM 5692 at time 2015.06.17_17:36_TAI
===== 103 =====
accept NOAA Active Region number 12371 and HARPNUM 5692 at time 2015.06.20_01:42_TAI
===== 104 =====
accept NOAA Active Region number 12371 and HARPNUM 5692 at time 2015.06.20_02:36_TAI
===== 105 =====
accept NOAA Active Region number 12371 and HARPNUM 5692 at time 2015.06.21_18:23_TAI
===== 106 =====
accept NOAA Active Region number 12371 and HARPNUM 5692 at time 2015.06.24_08:16_TAI
===== 107 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.20_09:48_TAI
===== 108 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.20_02:18_TAI
===== 109 =====
skip: there are no data for HARPNUM 5956 at time 2015.09.19_18:03_TAI
===== 110 =====
accept NOAA Active Region number 12423 and HARPNUM 5982 at time 2015.09.27_03:55_TAI
===== 111 =====
accept NOAA Active Region number 12445 and HARPNUM 6052 at time 2015.11.03_03:26_TAI
===== 112 =====
accept NOAA Active Region number 12443 and HARPNUM 6063 at time 2015.11.03_13:52_TAI
===== 113 =====
skip: there are no data for HARPNUM 6205 at time 2015.12.20_01:03_TAI
===== 114 =====
skip: there are no data for HARPNUM 6205 at time 2015.12.20_10:19_TAI
===== 115 =====
skip: latitude is out of range for HARPNUM 6206 at time 2015.12.22_00:40_TAI
===== 116 =====
skip: there are no data for HARPNUM 6206 at time 2015.12.27_12:45_TAI
===== 117 =====
accept NOAA Active Region number 12473 and HARPNUM 6206 at time 2016.01.01_00:11_TAI
===== 118 =====
accept NOAA Active Region number 12529 and HARPNUM 6483 at time 2016.04.17_00:29_TAI
===== 119 =====
accept NOAA Active Region number 12644 and HARPNUM 6972 at time 2017.03.31_21:48_TAI
===== 120 =====
accept NOAA Active Region number 12644 and HARPNUM 6972 at time 2017.04.01_08:02_TAI
===== 121 =====
accept NOAA Active Region number 12644 and HARPNUM 6972 at time 2017.04.01_18:38_TAI
===== 122 =====
accept NOAA Active Region number 12644 and HARPNUM 6972 at time 2017.04.02_01:05_TAI
===== 123 =====
accept NOAA Active Region number 12644 and HARPNUM 6972 at time 2017.04.02_14:29_TAI
===== 124 =====
accept NOAA Active Region number 12665 and HARPNUM 7075 at time 2017.07.13_02:09_TAI
===== 125 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.03_15:30_TAI
===== 126 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.03_18:22_TAI
===== 127 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.03_20:33_TAI
===== 128 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.05_12:02_TAI
===== 129 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.06_10:15_TAI
===== 130 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.06_14:36_TAI
===== 131 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.06_23:59_TAI
===== 132 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.07_03:43_TAI
===== 133 =====
skip: there are no data for HARPNUM 7115 at time 2017.09.07_07:49_TAI
===== 134 =====
skip: latitude is out of range for HARPNUM 7115 at time 2017.09.09_16:06_TAI
===== 135 =====
skip: there are no data for HARPNUM 7169 at time 2017.10.19_23:28_TAI
Here is the number of events associated with the positive class:
CME_data = positive_result[0]
positive_class = positive_result[1]
print("There are", len(CME_data), "CME events in the positive class.")
There are 77 CME events in the positive class.
Step 2: Gathering data for the negative class¶
To gather the examples for the negative class, we only need to:
Query the GOES database for all the M- and X-class flares during our time of interest, and
Select the ones that are not associated with a CME.
# select peak times that belong to both classes
all_peak_times = np.array([(listofresults[i]['peak_time'])
for i in range(len(listofresults))])
negative_class_possibilities = []
counter_positive = 0
counter_negative = 0
for i in range(len(listofresults)):
this_peak_time = all_peak_times[i]
if (this_peak_time in peak_times_donki):
counter_positive += 1
else:
counter_negative += 1
this_instance = [listofresults[i]['noaa_active_region'],
listofresults[i]['goes_class'], listofresults[i]['peak_time']]
negative_class_possibilities.append(this_instance)
print("There are", counter_positive, "events in the positive class.")
print("There are", counter_negative, "events in the negative class.")
There are 138 events in the positive class.
There are 655 events in the negative class.
Again, we compute times that are one day before the flare peak time and convert it into a string that JSOC can understand:
t_rec = np.array([(negative_class_possibilities[i][2] - timedelta(hours=timedelayvariable)
).strftime('%Y.%m.%d_%H:%M_TAI') for i in range(len(negative_class_possibilities))])
And again, we query the JSOC database to see if these data are present:
listofactiveregions = list(
negative_class_possibilities[i][0] for i in range(counter_negative))
listofgoesclasses = list(
negative_class_possibilities[i][1] for i in range(counter_negative))
negative_result = get_the_jsoc_data(counter_negative, t_rec)
===== 0 =====
accept NOAA Active Region number 11069 and HARPNUM 8 at time 2010.05.04_17:19_TAI
===== 1 =====
skip: there are no data for HARPNUM 54 at time 2010.06.11_00:57_TAI
===== 2 =====
accept NOAA Active Region number 11079 and HARPNUM 49 at time 2010.06.12_05:39_TAI
===== 3 =====
accept NOAA Active Region number 11093 and HARPNUM 115 at time 2010.08.06_18:24_TAI
===== 4 =====
accept NOAA Active Region number 11112 and HARPNUM 211 at time 2010.10.15_19:12_TAI
===== 5 =====
skip: latitude is out of range for HARPNUM 245 at time 2010.11.03_23:58_TAI
===== 6 =====
skip: latitude is out of range for HARPNUM 245 at time 2010.11.03_23:58_TAI
===== 7 =====
skip: latitude is out of range for HARPNUM 245 at time 2010.11.04_13:29_TAI
===== 8 =====
skip: latitude is out of range for HARPNUM 245 at time 2010.11.05_15:36_TAI
===== 9 =====
accept NOAA Active Region number 11149 and HARPNUM 345 at time 2011.01.27_01:03_TAI
===== 10 =====
accept NOAA Active Region number 11153 and HARPNUM 362 at time 2011.02.08_01:31_TAI
===== 11 =====
accept NOAA Active Region number 11158 and HARPNUM 377 at time 2011.02.12_17:38_TAI
===== 12 =====
accept NOAA Active Region number 11158 and HARPNUM 377 at time 2011.02.13_17:26_TAI
===== 13 =====
skip: there are no data for HARPNUM 1 at time 2011.02.15_01:39_TAI
===== 14 =====
accept NOAA Active Region number 11161 and HARPNUM 384 at time 2011.02.15_07:44_TAI
===== 15 =====
accept NOAA Active Region number 11158 and HARPNUM 377 at time 2011.02.15_14:25_TAI
===== 16 =====
accept NOAA Active Region number 11158 and HARPNUM 377 at time 2011.02.17_10:11_TAI
===== 17 =====
accept NOAA Active Region number 11162 and HARPNUM 384 at time 2011.02.17_10:26_TAI
===== 18 =====
accept NOAA Active Region number 11158 and HARPNUM 377 at time 2011.02.17_13:03_TAI
===== 19 =====
accept NOAA Active Region number 11162 and HARPNUM 384 at time 2011.02.17_14:08_TAI
===== 20 =====
accept NOAA Active Region number 11162 and HARPNUM 384 at time 2011.02.17_21:04_TAI
===== 21 =====
accept NOAA Active Region number 11164 and HARPNUM 393 at time 2011.02.27_12:52_TAI
===== 22 =====
accept NOAA Active Region number 11164 and HARPNUM 393 at time 2011.03.06_05:13_TAI
===== 23 =====
accept NOAA Active Region number 11165 and HARPNUM 394 at time 2011.03.06_07:54_TAI
===== 24 =====
accept NOAA Active Region number 11164 and HARPNUM 393 at time 2011.03.06_08:07_TAI
===== 25 =====
accept NOAA Active Region number 11164 and HARPNUM 393 at time 2011.03.06_09:20_TAI
===== 26 =====
accept NOAA Active Region number 11165 and HARPNUM 394 at time 2011.03.06_21:50_TAI
===== 27 =====
accept NOAA Active Region number 11165 and HARPNUM 394 at time 2011.03.07_02:29_TAI
===== 28 =====
skip: latitude is out of range for HARPNUM 394 at time 2011.03.07_10:44_TAI
===== 29 =====
skip: latitude is out of range for HARPNUM 394 at time 2011.03.07_18:28_TAI
===== 30 =====
skip: latitude is out of range for HARPNUM 394 at time 2011.03.07_20:16_TAI
===== 31 =====
accept NOAA Active Region number 11166 and HARPNUM 401 at time 2011.03.08_11:07_TAI
===== 32 =====
accept NOAA Active Region number 11166 and HARPNUM 401 at time 2011.03.08_14:02_TAI
===== 33 =====
accept NOAA Active Region number 11166 and HARPNUM 401 at time 2011.03.08_23:23_TAI
===== 34 =====
skip: there are no data for HARPNUM 1 at time 2011.03.09_22:41_TAI
===== 35 =====
accept NOAA Active Region number 11166 and HARPNUM 401 at time 2011.03.11_04:43_TAI
===== 36 =====
accept NOAA Active Region number 11169 and HARPNUM 407 at time 2011.03.13_19:52_TAI
===== 37 =====
accept NOAA Active Region number 11169 and HARPNUM 407 at time 2011.03.14_00:22_TAI
===== 38 =====
skip: latitude is out of range for HARPNUM 437 at time 2011.03.22_02:17_TAI
===== 39 =====
skip: latitude is out of range for HARPNUM 437 at time 2011.03.23_12:07_TAI
===== 40 =====
accept NOAA Active Region number 11176 and HARPNUM 437 at time 2011.03.24_23:22_TAI
===== 41 =====
accept NOAA Active Region number 11190 and HARPNUM 495 at time 2011.04.14_17:12_TAI
===== 42 =====
accept NOAA Active Region number 11195 and HARPNUM 514 at time 2011.04.21_04:57_TAI
===== 43 =====
accept NOAA Active Region number 11195 and HARPNUM 514 at time 2011.04.21_15:53_TAI
===== 44 =====
skip: latitude is out of range for HARPNUM 637 at time 2011.05.27_21:50_TAI
===== 45 =====
skip: latitude is out of range for HARPNUM 637 at time 2011.05.28_10:33_TAI
===== 46 =====
skip: latitude is out of range for HARPNUM 667 at time 2011.06.13_21:47_TAI
===== 47 =====
accept NOAA Active Region number 11260 and HARPNUM 746 at time 2011.07.26_16:07_TAI
===== 48 =====
accept NOAA Active Region number 11261 and HARPNUM 750 at time 2011.07.29_02:09_TAI
===== 49 =====
accept NOAA Active Region number 11261 and HARPNUM 750 at time 2011.08.01_06:19_TAI
===== 50 =====
accept NOAA Active Region number 11261 and HARPNUM 750 at time 2011.08.02_03:37_TAI
===== 51 =====
accept NOAA Active Region number 11263 and HARPNUM 753 at time 2011.08.02_04:32_TAI
===== 52 =====
skip: there are no data for HARPNUM 753 at time 2011.08.07_18:10_TAI
===== 53 =====
accept NOAA Active Region number 11263 and HARPNUM 753 at time 2011.08.08_03:54_TAI
===== 54 =====
accept NOAA Active Region number 11263 and HARPNUM 753 at time 2011.08.08_08:05_TAI
===== 55 =====
accept NOAA Active Region number 11286 and HARPNUM 814 at time 2011.09.03_11:45_TAI
===== 56 =====
accept NOAA Active Region number 11286 and HARPNUM 814 at time 2011.09.04_04:28_TAI
===== 57 =====
accept NOAA Active Region number 11286 and HARPNUM 814 at time 2011.09.04_07:58_TAI
===== 58 =====
accept NOAA Active Region number 11283 and HARPNUM 833 at time 2011.09.05_01:50_TAI
===== 59 =====
accept NOAA Active Region number 1283 and HARPNUM 833 at time 2011.09.05_22:20_TAI
===== 60 =====
accept NOAA Active Region number 11283 and HARPNUM 833 at time 2011.09.05_22:20_TAI
===== 61 =====
accept NOAA Active Region number 11283 and HARPNUM 833 at time 2011.09.07_15:46_TAI
===== 62 =====
skip: there are no data for HARPNUM 833 at time 2011.09.08_06:11_TAI
===== 63 =====
accept NOAA Active Region number 11283 and HARPNUM 833 at time 2011.09.08_12:49_TAI
===== 64 =====
accept NOAA Active Region number 11283 and HARPNUM 833 at time 2011.09.09_07:40_TAI
===== 65 =====
skip: there are no data for HARPNUM 878 at time 2011.09.20_12:23_TAI
===== 66 =====
skip: there are no data for HARPNUM 892 at time 2011.09.21_10:00_TAI
===== 67 =====
accept NOAA Active Region number 11295 and HARPNUM 856 at time 2011.09.22_01:59_TAI
===== 68 =====
accept NOAA Active Region number 11295 and HARPNUM 856 at time 2011.09.22_22:15_TAI
===== 69 =====
skip: latitude is out of range for HARPNUM 892 at time 2011.09.22_23:56_TAI
===== 70 =====
skip: there are no data for HARPNUM 892 at time 2011.09.23_09:40_TAI
===== 71 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.23_13:20_TAI
===== 72 =====
accept NOAA Active Region number 11295 and HARPNUM 856 at time 2011.09.23_16:59_TAI
===== 73 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.23_17:25_TAI
===== 74 =====
skip: there are no data for HARPNUM 892 at time 2011.09.23_18:15_TAI
===== 75 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.23_19:21_TAI
===== 76 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.23_20:36_TAI
===== 77 =====
skip: there are no data for HARPNUM 899 at time 2011.09.23_21:27_TAI
===== 78 =====
skip: there are no data for HARPNUM 899 at time 2011.09.23_23:58_TAI
===== 79 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.24_02:33_TAI
===== 80 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.24_04:50_TAI
===== 81 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.24_08:49_TAI
===== 82 =====
skip: there are no data for HARPNUM 899 at time 2011.09.24_09:35_TAI
===== 83 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.24_15:33_TAI
===== 84 =====
accept NOAA Active Region number 11303 and HARPNUM 899 at time 2011.09.24_16:58_TAI
===== 85 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.25_05:08_TAI
===== 86 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.25_14:46_TAI
===== 87 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.09.27_13:28_TAI
===== 88 =====
accept NOAA Active Region number 11305 and HARPNUM 902 at time 2011.09.29_19:06_TAI
===== 89 =====
skip: there are no data for HARPNUM 902 at time 2011.09.30_09:59_TAI
===== 90 =====
accept NOAA Active Region number 11305 and HARPNUM 902 at time 2011.10.01_00:50_TAI
===== 91 =====
accept NOAA Active Region number 11302 and HARPNUM 892 at time 2011.10.01_17:23_TAI
===== 92 =====
skip: there are no data for HARPNUM 1 at time 2011.10.19_03:25_TAI
===== 93 =====
accept NOAA Active Region number 11319 and HARPNUM 940 at time 2011.10.20_13:00_TAI
===== 94 =====
accept NOAA Active Region number 11314 and HARPNUM 940 at time 2011.10.21_11:10_TAI
===== 95 =====
skip: there are no data for HARPNUM 1 at time 2011.10.30_15:08_TAI
===== 96 =====
skip: there are no data for HARPNUM 1 at time 2011.10.30_18:08_TAI
===== 97 =====
skip: latitude is out of range for HARPNUM 1028 at time 2011.11.01_22:01_TAI
===== 98 =====
skip: latitude is out of range for HARPNUM 1028 at time 2011.11.02_11:11_TAI
===== 99 =====
skip: latitude is out of range for HARPNUM 1028 at time 2011.11.02_20:27_TAI
===== 100 =====
skip: latitude is out of range for HARPNUM 1028 at time 2011.11.02_23:36_TAI
===== 101 =====
accept NOAA Active Region number 11339 and HARPNUM 1028 at time 2011.11.03_20:40_TAI
===== 102 =====
accept NOAA Active Region number 11339 and HARPNUM 1028 at time 2011.11.04_03:35_TAI
===== 103 =====
accept NOAA Active Region number 11339 and HARPNUM 1028 at time 2011.11.04_11:21_TAI
===== 104 =====
accept NOAA Active Region number 11339 and HARPNUM 1028 at time 2011.11.04_20:38_TAI
===== 105 =====
accept NOAA Active Region number 11339 and HARPNUM 1028 at time 2011.11.05_01:03_TAI
===== 106 =====
accept NOAA Active Region number 11339 and HARPNUM 1028 at time 2011.11.05_06:35_TAI
===== 107 =====
accept NOAA Active Region number 11342 and HARPNUM 1041 at time 2011.11.08_13:35_TAI
===== 108 =====
skip: latitude is out of range for HARPNUM 1028 at time 2011.11.14_09:12_TAI
===== 109 =====
accept NOAA Active Region number 11346 and HARPNUM 1066 at time 2011.11.14_12:43_TAI
===== 110 =====
skip: latitude is out of range for HARPNUM 1028 at time 2011.11.14_22:35_TAI
===== 111 =====
skip: there are no data for HARPNUM 1209 at time 2011.12.24_18:16_TAI
===== 112 =====
accept NOAA Active Region number 11387 and HARPNUM 1209 at time 2011.12.25_02:27_TAI
===== 113 =====
accept NOAA Active Region number 11387 and HARPNUM 1209 at time 2011.12.25_20:30_TAI
===== 114 =====
skip: latitude is out of range for HARPNUM 1256 at time 2011.12.28_13:50_TAI
===== 115 =====
skip: latitude is out of range for HARPNUM 1256 at time 2011.12.28_21:51_TAI
===== 116 =====
accept NOAA Active Region number 11389 and HARPNUM 1256 at time 2011.12.29_03:09_TAI
===== 117 =====
accept NOAA Active Region number 11389 and HARPNUM 1256 at time 2011.12.30_13:15_TAI
===== 118 =====
accept NOAA Active Region number 11389 and HARPNUM 1256 at time 2011.12.30_16:26_TAI
===== 119 =====
skip: there are no data for HARPNUM 1321 at time 2012.01.13_13:18_TAI
===== 120 =====
accept NOAA Active Region number 11401 and HARPNUM 1321 at time 2012.01.16_04:53_TAI
===== 121 =====
skip: there are no data for HARPNUM 1321 at time 2012.01.17_19:12_TAI
===== 122 =====
skip: there are no data for HARPNUM 1321 at time 2012.01.18_16:05_TAI
===== 123 =====
accept NOAA Active Region number 11410 and HARPNUM 1350 at time 2012.02.05_20:00_TAI
===== 124 =====
skip: there are no data for HARPNUM 1449 at time 2012.03.01_17:46_TAI
===== 125 =====
skip: latitude is out of range for HARPNUM 1449 at time 2012.03.03_10:52_TAI
===== 126 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.04_19:16_TAI
===== 127 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.04_19:30_TAI
===== 128 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.04_22:34_TAI
===== 129 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.05_00:28_TAI
===== 130 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.05_01:44_TAI
===== 131 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.05_04:05_TAI
===== 132 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.05_07:55_TAI
===== 133 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.05_12:41_TAI
===== 134 =====
skip: there are no data for HARPNUM 1 at time 2012.03.05_21:11_TAI
===== 135 =====
accept NOAA Active Region number 11429 and HARPNUM 1449 at time 2012.03.05_22:53_TAI
===== 136 =====
accept NOAA Active Region number 11432 and HARPNUM 1461 at time 2012.03.13_15:21_TAI
===== 137 =====
skip: there are no data for HARPNUM 1461 at time 2012.03.14_07:52_TAI
===== 138 =====
accept NOAA Active Region number 11434 and HARPNUM 1464 at time 2012.03.16_20:39_TAI
===== 139 =====
skip: there are no data for HARPNUM 1500 at time 2012.03.22_19:40_TAI
===== 140 =====
skip: there are no data for HARPNUM 1582 at time 2012.04.15_17:45_TAI
===== 141 =====
accept NOAA Active Region number 11466 and HARPNUM 1603 at time 2012.04.26_08:24_TAI
===== 142 =====
skip: latitude is out of range for HARPNUM 1638 at time 2012.05.04_13:23_TAI
===== 143 =====
skip: latitude is out of range for HARPNUM 1638 at time 2012.05.04_23:01_TAI
===== 144 =====
skip: there are no data for HARPNUM 1638 at time 2012.05.05_01:18_TAI
===== 145 =====
skip: there are no data for HARPNUM 1638 at time 2012.05.05_17:47_TAI
===== 146 =====
accept NOAA Active Region number 11471 and HARPNUM 1621 at time 2012.05.06_14:31_TAI
===== 147 =====
accept NOAA Active Region number 11476 and HARPNUM 1638 at time 2012.05.07_13:08_TAI
===== 148 =====
accept NOAA Active Region number 11476 and HARPNUM 1638 at time 2012.05.08_12:32_TAI
===== 149 =====
accept NOAA Active Region number 11476 and HARPNUM 1638 at time 2012.05.08_14:08_TAI
===== 150 =====
accept NOAA Active Region number 11476 and HARPNUM 1638 at time 2012.05.08_21:05_TAI
===== 151 =====
accept NOAA Active Region number 11476 and HARPNUM 1638 at time 2012.05.09_04:18_TAI
===== 152 =====
accept NOAA Active Region number 11476 and HARPNUM 1638 at time 2012.05.09_20:26_TAI
===== 153 =====
skip: there are no data for HARPNUM 1722 at time 2012.06.02_17:55_TAI
===== 154 =====
accept NOAA Active Region number 11494 and HARPNUM 1724 at time 2012.06.05_20:06_TAI
===== 155 =====
skip: latitude is out of range for HARPNUM 1750 at time 2012.06.08_11:32_TAI
===== 156 =====
skip: latitude is out of range for HARPNUM 1750 at time 2012.06.08_16:53_TAI
===== 157 =====
skip: latitude is out of range for HARPNUM 1750 at time 2012.06.09_06:45_TAI
===== 158 =====
skip: latitude is out of range for HARPNUM 1806 at time 2012.06.27_16:12_TAI
===== 159 =====
accept NOAA Active Region number 11513 and HARPNUM 1806 at time 2012.06.28_09:20_TAI
===== 160 =====
accept NOAA Active Region number 11513 and HARPNUM 1806 at time 2012.06.29_12:52_TAI
===== 161 =====
accept NOAA Active Region number 11513 and HARPNUM 1806 at time 2012.06.29_18:32_TAI
===== 162 =====
accept NOAA Active Region number 11513 and HARPNUM 1806 at time 2012.06.30_19:18_TAI
===== 163 =====
accept NOAA Active Region number 11513 and HARPNUM 1806 at time 2012.07.01_00:35_TAI
===== 164 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.01_10:52_TAI
===== 165 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.01_20:07_TAI
===== 166 =====
skip: there are no data for HARPNUM 1807 at time 2012.07.01_23:56_TAI
===== 167 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.03_04:37_TAI
===== 168 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.03_09:55_TAI
===== 169 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.03_12:24_TAI
===== 170 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.03_14:40_TAI
===== 171 =====
accept NOAA Active Region number 11513 and HARPNUM 1806 at time 2012.07.03_16:39_TAI
===== 172 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.03_22:09_TAI
===== 173 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.03_23:55_TAI
===== 174 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_01:10_TAI
===== 175 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_02:42_TAI
===== 176 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_03:36_TAI
===== 177 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_06:58_TAI
===== 178 =====
skip: latitude is out of range for HARPNUM 1834 at time 2012.07.04_07:45_TAI
===== 179 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_10:48_TAI
===== 180 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_11:44_TAI
===== 181 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_13:18_TAI
===== 182 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_20:14_TAI
===== 183 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.04_21:45_TAI
===== 184 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.05_01:40_TAI
===== 185 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.05_02:51_TAI
===== 186 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.05_08:23_TAI
===== 187 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.05_10:29_TAI
===== 188 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.05_13:30_TAI
===== 189 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.05_18:55_TAI
===== 190 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.06_03:15_TAI
===== 191 =====
skip: latitude is out of range for HARPNUM 1834 at time 2012.07.06_08:28_TAI
===== 192 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.06_11:03_TAI
===== 193 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.07_05:46_TAI
===== 194 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.07_09:53_TAI
===== 195 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.07_12:10_TAI
===== 196 =====
accept NOAA Active Region number 11515 and HARPNUM 1807 at time 2012.07.07_16:32_TAI
===== 197 =====
accept NOAA Active Region number 11520 and HARPNUM 1834 at time 2012.07.08_23:07_TAI
===== 198 =====
accept NOAA Active Region number 11520 and HARPNUM 1834 at time 2012.07.09_05:14_TAI
===== 199 =====
accept NOAA Active Region number 11520 and HARPNUM 1834 at time 2012.07.09_06:27_TAI
===== 200 =====
accept NOAA Active Region number 11521 and HARPNUM 1834 at time 2012.07.13_04:58_TAI
===== 201 =====
skip: there are no data for HARPNUM 1879 at time 2012.07.26_17:26_TAI
===== 202 =====
accept NOAA Active Region number 11532 and HARPNUM 1879 at time 2012.07.28_06:22_TAI
===== 203 =====
accept NOAA Active Region number 11536 and HARPNUM 1879 at time 2012.07.29_15:48_TAI
===== 204 =====
skip: there are no data for HARPNUM 1930 at time 2012.08.05_04:38_TAI
===== 205 =====
accept NOAA Active Region number 11540 and HARPNUM 1907 at time 2012.08.10_12:20_TAI
===== 206 =====
skip: there are no data for HARPNUM 1946 at time 2012.08.16_13:19_TAI
===== 207 =====
skip: there are no data for HARPNUM 1946 at time 2012.08.16_17:20_TAI
===== 208 =====
skip: there are no data for HARPNUM 1946 at time 2012.08.17_01:02_TAI
===== 209 =====
skip: there are no data for HARPNUM 1946 at time 2012.08.17_03:23_TAI
===== 210 =====
skip: there are no data for HARPNUM 1946 at time 2012.08.17_16:07_TAI
===== 211 =====
skip: latitude is out of range for HARPNUM 1946 at time 2012.08.17_22:54_TAI
===== 212 =====
skip: latitude is out of range for HARPNUM 1946 at time 2012.08.17_23:22_TAI
===== 213 =====
skip: there are no data for HARPNUM 1996 at time 2012.08.29_12:11_TAI
===== 214 =====
accept NOAA Active Region number 11560 and HARPNUM 1993 at time 2012.09.05_04:13_TAI
===== 215 =====
skip: there are no data for HARPNUM 1999 at time 2012.09.07_17:59_TAI
===== 216 =====
accept NOAA Active Region number 11564 and HARPNUM 1999 at time 2012.09.08_22:36_TAI
===== 217 =====
accept NOAA Active Region number 11583 and HARPNUM 2040 at time 2012.09.29_04:33_TAI
===== 218 =====
skip: there are no data for HARPNUM 1 at time 2012.10.07_11:17_TAI
===== 219 =====
skip: there are no data for HARPNUM 1 at time 2012.10.08_23:31_TAI
===== 220 =====
skip: there are no data for HARPNUM 1 at time 2012.10.09_05:04_TAI
===== 221 =====
skip: there are no data for HARPNUM 2137 at time 2012.10.19_18:14_TAI
===== 222 =====
skip: latitude is out of range for HARPNUM 2137 at time 2012.10.20_20:03_TAI
===== 223 =====
skip: latitude is out of range for HARPNUM 2137 at time 2012.10.21_18:51_TAI
===== 224 =====
skip: there are no data for HARPNUM 2137 at time 2012.10.22_03:17_TAI
===== 225 =====
skip: there are no data for HARPNUM 2193 at time 2012.11.10_02:33_TAI
===== 226 =====
accept NOAA Active Region number 11613 and HARPNUM 2191 at time 2012.11.11_23:28_TAI
===== 227 =====
accept NOAA Active Region number 11613 and HARPNUM 2191 at time 2012.11.12_02:04_TAI
===== 228 =====
accept NOAA Active Region number 11613 and HARPNUM 2191 at time 2012.11.12_05:50_TAI
===== 229 =====
accept NOAA Active Region number 11613 and HARPNUM 2191 at time 2012.11.12_20:54_TAI
===== 230 =====
accept NOAA Active Region number 11613 and HARPNUM 2191 at time 2012.11.13_04:04_TAI
===== 231 =====
skip: there are no data for HARPNUM 1 at time 2012.11.19_12:41_TAI
===== 232 =====
accept NOAA Active Region number 11618 and HARPNUM 2220 at time 2012.11.19_19:28_TAI
===== 233 =====
accept NOAA Active Region number 11618 and HARPNUM 2220 at time 2012.11.20_06:56_TAI
===== 234 =====
accept NOAA Active Region number 11618 and HARPNUM 2220 at time 2012.11.26_15:57_TAI
===== 235 =====
accept NOAA Active Region number 11620 and HARPNUM 2227 at time 2012.11.26_21:26_TAI
===== 236 =====
accept NOAA Active Region number 11620 and HARPNUM 2227 at time 2012.11.27_21:36_TAI
===== 237 =====
skip: there are no data for HARPNUM 2362 at time 2013.01.04_09:31_TAI
===== 238 =====
accept NOAA Active Region number 11654 and HARPNUM 2372 at time 2013.01.10_09:11_TAI
===== 239 =====
accept NOAA Active Region number 11654 and HARPNUM 2372 at time 2013.01.10_15:07_TAI
===== 240 =====
accept NOAA Active Region number 11652 and HARPNUM 2362 at time 2013.01.12_00:50_TAI
===== 241 =====
accept NOAA Active Region number 11652 and HARPNUM 2362 at time 2013.01.12_08:38_TAI
===== 242 =====
accept NOAA Active Region number 11675 and HARPNUM 2491 at time 2013.02.16_15:50_TAI
===== 243 =====
skip: there are no data for HARPNUM 2519 at time 2013.03.04_07:54_TAI
===== 244 =====
skip: there are no data for HARPNUM 2546 at time 2013.03.14_06:58_TAI
===== 245 =====
accept NOAA Active Region number 11692 and HARPNUM 2546 at time 2013.03.20_22:04_TAI
===== 246 =====
skip: there are no data for HARPNUM 2635 at time 2013.04.04_17:48_TAI
===== 247 =====
accept NOAA Active Region number 11718 and HARPNUM 2636 at time 2013.04.11_20:38_TAI
===== 248 =====
accept NOAA Active Region number 11726 and HARPNUM 2673 at time 2013.04.21_10:29_TAI
===== 249 =====
accept NOAA Active Region number 11731 and HARPNUM 2693 at time 2013.05.01_05:10_TAI
===== 250 =====
accept NOAA Active Region number 11731 and HARPNUM 2693 at time 2013.05.02_16:55_TAI
===== 251 =====
skip: there are no data for HARPNUM 2716 at time 2013.05.02_17:32_TAI
===== 252 =====
skip: there are no data for HARPNUM 2716 at time 2013.05.04_17:56_TAI
===== 253 =====
skip: there are no data for HARPNUM 2739 at time 2013.05.09_00:57_TAI
===== 254 =====
skip: there are no data for HARPNUM 2739 at time 2013.05.09_12:56_TAI
===== 255 =====
skip: there are no data for HARPNUM 2748 at time 2013.05.11_20:32_TAI
===== 256 =====
skip: there are no data for HARPNUM 2748 at time 2013.05.11_22:44_TAI
===== 257 =====
skip: there are no data for HARPNUM 2748 at time 2013.05.12_12:03_TAI
===== 258 =====
accept NOAA Active Region number 11748 and HARPNUM 2748 at time 2013.05.15_21:53_TAI
===== 259 =====
accept NOAA Active Region number 11748 and HARPNUM 2748 at time 2013.05.16_08:57_TAI
===== 260 =====
skip: there are no data for HARPNUM 2760 at time 2013.05.19_05:25_TAI
===== 261 =====
accept NOAA Active Region number 11760 and HARPNUM 2809 at time 2013.05.30_20:00_TAI
===== 262 =====
accept NOAA Active Region number 11762 and HARPNUM 2790 at time 2013.06.04_08:57_TAI
===== 263 =====
skip: latitude is out of range for HARPNUM 2790 at time 2013.06.06_22:49_TAI
===== 264 =====
skip: latitude is out of range for HARPNUM 2878 at time 2013.06.20_03:14_TAI
===== 265 =====
skip: latitude is out of range for HARPNUM 2887 at time 2013.06.22_20:56_TAI
===== 266 =====
skip: latitude is out of range for HARPNUM 2920 at time 2013.07.02_07:08_TAI
===== 267 =====
accept NOAA Active Region number 11817 and HARPNUM 3048 at time 2013.08.11_10:41_TAI
===== 268 =====
accept NOAA Active Region number 11818 and HARPNUM 3056 at time 2013.08.16_18:24_TAI
===== 269 =====
accept NOAA Active Region number 11818 and HARPNUM 3056 at time 2013.08.16_19:33_TAI
===== 270 =====
skip: latitude is out of range for HARPNUM 3263 at time 2013.10.08_01:48_TAI
===== 271 =====
skip: there are no data for HARPNUM 1 at time 2013.10.10_07:25_TAI
===== 272 =====
accept NOAA Active Region number 11865 and HARPNUM 3263 at time 2013.10.12_00:43_TAI
===== 273 =====
accept NOAA Active Region number 11865 and HARPNUM 3263 at time 2013.10.14_08:38_TAI
===== 274 =====
accept NOAA Active Region number 11865 and HARPNUM 3263 at time 2013.10.14_23:36_TAI
===== 275 =====
accept NOAA Active Region number 11861 and HARPNUM 3258 at time 2013.10.16_15:41_TAI
===== 276 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.21_00:22_TAI
===== 277 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.21_15:20_TAI
===== 278 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.22_20:53_TAI
===== 279 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.22_23:43_TAI
===== 280 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.23_00:08_TAI
===== 281 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.23_10:09_TAI
===== 282 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.23_10:33_TAI
===== 283 =====
skip: latitude is out of range for HARPNUM 3311 at time 2013.10.24_03:02_TAI
===== 284 =====
skip: latitude is out of range for HARPNUM 3311 at time 2013.10.24_10:12_TAI
===== 285 =====
skip: there are no data for HARPNUM 3311 at time 2013.10.24_17:09_TAI
===== 286 =====
skip: there are no data for HARPNUM 3311 at time 2013.10.24_19:21_TAI
===== 287 =====
skip: latitude is out of range for HARPNUM 3311 at time 2013.10.24_20:58_TAI
===== 288 =====
skip: there are no data for HARPNUM 3311 at time 2013.10.25_06:06_TAI
===== 289 =====
skip: latitude is out of range for HARPNUM 3311 at time 2013.10.25_09:37_TAI
===== 290 =====
skip: latitude is out of range for HARPNUM 3311 at time 2013.10.25_11:17_TAI
===== 291 =====
accept NOAA Active Region number 11882 and HARPNUM 3311 at time 2013.10.25_19:53_TAI
===== 292 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.26_12:48_TAI
===== 293 =====
accept NOAA Active Region number 11877 and HARPNUM 3295 at time 2013.10.27_11:53_TAI
===== 294 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.27_14:05_TAI
===== 295 =====
accept NOAA Active Region number 11882 and HARPNUM 3311 at time 2013.10.27_15:01_TAI
===== 296 =====
accept NOAA Active Region number 11882 and HARPNUM 3311 at time 2013.10.27_15:15_TAI
===== 297 =====
accept NOAA Active Region number 11875 and HARPNUM 3291 at time 2013.10.27_20:57_TAI
===== 298 =====
skip: latitude is out of range for HARPNUM 3295 at time 2013.10.30_13:51_TAI
===== 299 =====
accept NOAA Active Region number 11884 and HARPNUM 3321 at time 2013.10.31_19:53_TAI
===== 300 =====
accept NOAA Active Region number 11884 and HARPNUM 3321 at time 2013.11.01_22:21_TAI
===== 301 =====
accept NOAA Active Region number 11884 and HARPNUM 3321 at time 2013.11.02_05:22_TAI
===== 302 =====
accept NOAA Active Region number 11890 and HARPNUM 3341 at time 2013.11.04_08:18_TAI
===== 303 =====
skip: there are no data for HARPNUM 3341 at time 2013.11.04_18:13_TAI
===== 304 =====
accept NOAA Active Region number 11890 and HARPNUM 3341 at time 2013.11.04_22:12_TAI
===== 305 =====
accept NOAA Active Region number 11890 and HARPNUM 3341 at time 2013.11.05_13:46_TAI
===== 306 =====
skip: there are no data for HARPNUM 1 at time 2013.11.06_00:02_TAI
===== 307 =====
accept NOAA Active Region number 11890 and HARPNUM 3341 at time 2013.11.06_03:40_TAI
===== 308 =====
accept NOAA Active Region number 11890 and HARPNUM 3341 at time 2013.11.06_14:25_TAI
===== 309 =====
accept NOAA Active Region number 11891 and HARPNUM 3344 at time 2013.11.07_09:28_TAI
===== 310 =====
skip: latitude is out of range for HARPNUM 3366 at time 2013.11.10_11:18_TAI
===== 311 =====
skip: there are no data for HARPNUM 3366 at time 2013.11.12_15:20_TAI
===== 312 =====
accept NOAA Active Region number 11899 and HARPNUM 3376 at time 2013.11.14_02:29_TAI
===== 313 =====
accept NOAA Active Region number 11900 and HARPNUM 3364 at time 2013.11.15_04:53_TAI
===== 314 =====
accept NOAA Active Region number 11900 and HARPNUM 3364 at time 2013.11.15_07:49_TAI
===== 315 =====
accept NOAA Active Region number 11900 and HARPNUM 3364 at time 2013.11.16_05:10_TAI
===== 316 =====
skip: latitude is out of range for HARPNUM 3364 at time 2013.11.20_11:11_TAI
===== 317 =====
accept NOAA Active Region number 11904 and HARPNUM 3376 at time 2013.11.22_02:32_TAI
===== 318 =====
accept NOAA Active Region number 11904 and HARPNUM 3376 at time 2013.11.22_12:57_TAI
===== 319 =====
accept NOAA Active Region number 11909 and HARPNUM 3437 at time 2013.12.06_07:29_TAI
===== 320 =====
skip: latitude is out of range for HARPNUM 3520 at time 2013.12.18_23:19_TAI
===== 321 =====
skip: latitude is out of range for HARPNUM 3520 at time 2013.12.19_11:57_TAI
===== 322 =====
accept NOAA Active Region number 11928 and HARPNUM 3497 at time 2013.12.21_08:11_TAI
===== 323 =====
accept NOAA Active Region number 11928 and HARPNUM 3497 at time 2013.12.21_08:37_TAI
===== 324 =====
accept NOAA Active Region number 11934 and HARPNUM 3520 at time 2013.12.21_14:38_TAI
===== 325 =====
accept NOAA Active Region number 11928 and HARPNUM 3497 at time 2013.12.21_15:12_TAI
===== 326 =====
accept NOAA Active Region number 11928 and HARPNUM 3497 at time 2013.12.21_22:08_TAI
===== 327 =====
accept NOAA Active Region number 11928 and HARPNUM 3497 at time 2013.12.22_00:03_TAI
===== 328 =====
accept NOAA Active Region number 11928 and HARPNUM 3497 at time 2013.12.22_09:06_TAI
===== 329 =====
accept NOAA Active Region number 11936 and HARPNUM 3535 at time 2013.12.28_07:56_TAI
===== 330 =====
accept NOAA Active Region number 11936 and HARPNUM 3535 at time 2013.12.30_21:58_TAI
===== 331 =====
accept NOAA Active Region number 11936 and HARPNUM 3535 at time 2013.12.31_18:52_TAI
===== 332 =====
skip: latitude is out of range for HARPNUM 3563 at time 2014.01.01_02:33_TAI
===== 333 =====
skip: latitude is out of range for HARPNUM 3563 at time 2014.01.01_22:18_TAI
===== 334 =====
skip: latitude is out of range for HARPNUM 3563 at time 2014.01.02_12:50_TAI
===== 335 =====
skip: latitude is out of range for HARPNUM 3563 at time 2014.01.02_21:14_TAI
===== 336 =====
accept NOAA Active Region number 11944 and HARPNUM 3563 at time 2014.01.03_10:25_TAI
===== 337 =====
accept NOAA Active Region number 11944 and HARPNUM 3563 at time 2014.01.03_10:25_TAI
===== 338 =====
accept NOAA Active Region number 11946 and HARPNUM 3580 at time 2014.01.06_03:53_TAI
===== 339 =====
accept NOAA Active Region number 11944 and HARPNUM 3563 at time 2014.01.06_10:13_TAI
===== 340 =====
accept NOAA Active Region number 11944 and HARPNUM 3563 at time 2014.01.12_21:51_TAI
===== 341 =====
skip: there are no data for HARPNUM 1 at time 2014.01.26_01:22_TAI
===== 342 =====
skip: there are no data for HARPNUM 1 at time 2014.01.26_02:11_TAI
===== 343 =====
skip: there are no data for HARPNUM 3686 at time 2014.01.26_22:10_TAI
===== 344 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.27_04:09_TAI
===== 345 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.27_07:31_TAI
===== 346 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.27_11:38_TAI
===== 347 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.27_12:46_TAI
===== 348 =====
skip: there are no data for HARPNUM 1 at time 2014.01.27_12:46_TAI
===== 349 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.27_19:40_TAI
===== 350 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.27_22:16_TAI
===== 351 =====
skip: latitude is out of range for HARPNUM 3686 at time 2014.01.29_06:39_TAI
===== 352 =====
skip: there are no data for HARPNUM 3688 at time 2014.01.30_15:42_TAI
===== 353 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.01.31_01:25_TAI
===== 354 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.01.31_07:23_TAI
===== 355 =====
accept NOAA Active Region number 11968 and HARPNUM 3688 at time 2014.02.01_06:34_TAI
===== 356 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.01_08:20_TAI
===== 357 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.01_09:31_TAI
===== 358 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.01_14:06_TAI
===== 359 =====
skip: there are no data for HARPNUM 1 at time 2014.02.01_16:29_TAI
===== 360 =====
skip: there are no data for HARPNUM 3686 at time 2014.02.01_18:11_TAI
===== 361 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.01_22:04_TAI
===== 362 =====
accept NOAA Active Region number 11968 and HARPNUM 3688 at time 2014.02.03_01:23_TAI
===== 363 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.03_04:00_TAI
===== 364 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.03_09:49_TAI
===== 365 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.03_16:02_TAI
===== 366 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.04_16:20_TAI
===== 367 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.05_23:05_TAI
===== 368 =====
accept NOAA Active Region number 11967 and HARPNUM 3686 at time 2014.02.06_04:56_TAI
===== 369 =====
accept NOAA Active Region number 11968 and HARPNUM 3688 at time 2014.02.06_10:29_TAI
===== 370 =====
skip: there are no data for HARPNUM 1 at time 2014.02.08_16:17_TAI
===== 371 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.10_16:51_TAI
===== 372 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.11_04:25_TAI
===== 373 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.11_06:58_TAI
===== 374 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.11_15:51_TAI
===== 375 =====
skip: there are no data for HARPNUM 1 at time 2014.02.12_01:40_TAI
===== 376 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.12_02:51_TAI
===== 377 =====
skip: there are no data for HARPNUM 3721 at time 2014.02.12_06:07_TAI
===== 378 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.12_08:12_TAI
===== 379 =====
skip: there are no data for HARPNUM 1 at time 2014.02.12_15:57_TAI
===== 380 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.13_02:57_TAI
===== 381 =====
skip: there are no data for HARPNUM 3721 at time 2014.02.13_12:40_TAI
===== 382 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.13_13:28_TAI
===== 383 =====
accept NOAA Active Region number 11974 and HARPNUM 3721 at time 2014.02.13_16:39_TAI
===== 384 =====
accept NOAA Active Region number 11977 and HARPNUM 3740 at time 2014.02.15_09:26_TAI
===== 385 =====
accept NOAA Active Region number 11976 and HARPNUM 3730 at time 2014.02.19_07:56_TAI
===== 386 =====
skip: there are no data for HARPNUM 1 at time 2014.02.22_06:10_TAI
===== 387 =====
skip: there are no data for HARPNUM 3793 at time 2014.02.23_11:17_TAI
===== 388 =====
skip: there are no data for HARPNUM 3793 at time 2014.02.23_12:05_TAI
===== 389 =====
accept NOAA Active Region number 11982 and HARPNUM 3766 at time 2014.02.25_15:01_TAI
===== 390 =====
accept NOAA Active Region number 11991 and HARPNUM 3804 at time 2014.02.27_00:48_TAI
===== 391 =====
skip: latitude is out of range for HARPNUM 3766 at time 2014.02.28_13:33_TAI
===== 392 =====
accept NOAA Active Region number 11986 and HARPNUM 3779 at time 2014.03.01_23:19_TAI
===== 393 =====
accept NOAA Active Region number 11989 and HARPNUM 3784 at time 2014.03.02_15:58_TAI
===== 394 =====
accept NOAA Active Region number 11991 and HARPNUM 3804 at time 2014.03.04_02:10_TAI
===== 395 =====
skip: latitude is out of range for HARPNUM 3836 at time 2014.03.07_23:41_TAI
===== 396 =====
skip: latitude is out of range for HARPNUM 3836 at time 2014.03.08_13:58_TAI
===== 397 =====
accept NOAA Active Region number 12002 and HARPNUM 3836 at time 2014.03.08_20:28_TAI
===== 398 =====
accept NOAA Active Region number 12002 and HARPNUM 3836 at time 2014.03.09_00:26_TAI
===== 399 =====
accept NOAA Active Region number 12002 and HARPNUM 3836 at time 2014.03.09_04:08_TAI
===== 400 =====
accept NOAA Active Region number 12002 and HARPNUM 3836 at time 2014.03.09_15:28_TAI
===== 401 =====
accept NOAA Active Region number 11996 and HARPNUM 3813 at time 2014.03.09_23:00_TAI
===== 402 =====
accept NOAA Active Region number 11996 and HARPNUM 3813 at time 2014.03.10_03:50_TAI
===== 403 =====
skip: latitude is out of range for HARPNUM 3804 at time 2014.03.10_12:07_TAI
===== 404 =====
accept NOAA Active Region number 11996 and HARPNUM 3813 at time 2014.03.11_11:05_TAI
===== 405 =====
accept NOAA Active Region number 11996 and HARPNUM 3813 at time 2014.03.11_22:34_TAI
===== 406 =====
skip: latitude is out of range for HARPNUM 3813 at time 2014.03.12_19:19_TAI
===== 407 =====
accept NOAA Active Region number 12010 and HARPNUM 3856 at time 2014.03.19_03:56_TAI
===== 408 =====
skip: there are no data for HARPNUM 3877 at time 2014.03.21_07:02_TAI
===== 409 =====
accept NOAA Active Region number 12017 and HARPNUM 3894 at time 2014.03.27_19:18_TAI
===== 410 =====
accept NOAA Active Region number 12017 and HARPNUM 3894 at time 2014.03.27_23:51_TAI
===== 411 =====
accept NOAA Active Region number 12017 and HARPNUM 3894 at time 2014.03.29_11:55_TAI
===== 412 =====
accept NOAA Active Region number 12014 and HARPNUM 3879 at time 2014.03.30_08:07_TAI
===== 413 =====
accept NOAA Active Region number 12035 and HARPNUM 4000 at time 2014.04.15_19:59_TAI
===== 414 =====
accept NOAA Active Region number 12056 and HARPNUM 4097 at time 2014.05.07_10:07_TAI
===== 415 =====
accept NOAA Active Region number 12065 and HARPNUM 4138 at time 2014.05.23_18:35_TAI
===== 416 =====
accept NOAA Active Region number 12077 and HARPNUM 4186 at time 2014.06.02_04:09_TAI
===== 417 =====
accept NOAA Active Region number 12080 and HARPNUM 4197 at time 2014.06.05_19:31_TAI
===== 418 =====
accept NOAA Active Region number 12080 and HARPNUM 4197 at time 2014.06.10_05:34_TAI
===== 419 =====
skip: latitude is out of range for HARPNUM 4225 at time 2014.06.10_08:09_TAI
===== 420 =====
skip: latitude is out of range for HARPNUM 4225 at time 2014.06.10_09:06_TAI
===== 421 =====
skip: latitude is out of range for HARPNUM 4225 at time 2014.06.10_21:03_TAI
===== 422 =====
skip: latitude is out of range for HARPNUM 4225 at time 2014.06.11_04:21_TAI
===== 423 =====
accept NOAA Active Region number 12085 and HARPNUM 4197 at time 2014.06.11_09:37_TAI
===== 424 =====
skip: latitude is out of range for HARPNUM 4225 at time 2014.06.11_10:21_TAI
===== 425 =====
skip: there are no data for HARPNUM 4225 at time 2014.06.11_18:13_TAI
===== 426 =====
accept NOAA Active Region number 12089 and HARPNUM 4231 at time 2014.06.11_20:03_TAI
===== 427 =====
skip: latitude is out of range for HARPNUM 4225 at time 2014.06.11_21:13_TAI
===== 428 =====
skip: latitude is out of range for HARPNUM 4225 at time 2014.06.12_07:56_TAI
===== 429 =====
skip: there are no data for HARPNUM 1 at time 2014.06.13_19:29_TAI
===== 430 =====
accept NOAA Active Region number 12085 and HARPNUM 4197 at time 2014.06.14_11:39_TAI
===== 431 =====
accept NOAA Active Region number 12087 and HARPNUM 4225 at time 2014.06.15_00:01_TAI
===== 432 =====
accept NOAA Active Region number 12113 and HARPNUM 4344 at time 2014.07.08_00:26_TAI
===== 433 =====
accept NOAA Active Region number 12106 and HARPNUM 4294 at time 2014.07.09_22:34_TAI
===== 434 =====
accept NOAA Active Region number 12130 and HARPNUM 4396 at time 2014.07.30_11:14_TAI
===== 435 =====
skip: there are no data for HARPNUM 4396 at time 2014.07.31_14:48_TAI
===== 436 =====
skip: there are no matching HARPNUMs for 12149
===== 437 =====
skip: there are no matching HARPNUMs for 12151
===== 438 =====
skip: there are no matching HARPNUMs for 12155
===== 439 =====
skip: there are no matching HARPNUMs for 12157
===== 440 =====
skip: there are no matching HARPNUMs for 12158
===== 441 =====
skip: there are no matching HARPNUMs for 12166
===== 442 =====
skip: there are no matching HARPNUMs for 12166
===== 443 =====
skip: there are no matching HARPNUMs for 12169
===== 444 =====
skip: there are no data for HARPNUM 1 at time 2014.09.26_08:37_TAI
===== 445 =====
skip: there are no matching HARPNUMs for 12173
===== 446 =====
skip: there are no matching HARPNUMs for 12172
===== 447 =====
accept NOAA Active Region number 12182 and HARPNUM 4639 at time 2014.10.08_01:43_TAI
===== 448 =====
accept NOAA Active Region number 12182 and HARPNUM 4639 at time 2014.10.08_01:58_TAI
===== 449 =====
accept NOAA Active Region number 12182 and HARPNUM 4639 at time 2014.10.08_06:59_TAI
===== 450 =====
skip: there are no data for HARPNUM 1 at time 2014.10.13_18:37_TAI
===== 451 =====
skip: there are no data for HARPNUM 1 at time 2014.10.13_21:21_TAI
===== 452 =====
skip: latitude is out of range for HARPNUM 4698 at time 2014.10.17_07:58_TAI
===== 453 =====
skip: latitude is out of range for HARPNUM 4698 at time 2014.10.18_05:03_TAI
===== 454 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.19_09:11_TAI
===== 455 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.19_16:37_TAI
===== 456 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.19_19:02_TAI
===== 457 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.19_20:04_TAI
===== 458 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.19_22:55_TAI
===== 459 =====
skip: there are no data for HARPNUM 1 at time 2014.10.20_13:38_TAI
===== 460 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.21_01:59_TAI
===== 461 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.21_05:17_TAI
===== 462 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.21_14:28_TAI
===== 463 =====
skip: there are no data for HARPNUM 1 at time 2014.10.21_15:57_TAI
===== 464 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.22_09:50_TAI
===== 465 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.23_21:41_TAI
===== 466 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.24_17:08_TAI
===== 467 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.25_10:56_TAI
===== 468 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.25_17:17_TAI
===== 469 =====
skip: there are no data for HARPNUM 4698 at time 2014.10.25_18:15_TAI
===== 470 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.25_18:49_TAI
===== 471 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.25_20:21_TAI
===== 472 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.26_00:34_TAI
===== 473 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.26_02:02_TAI
===== 474 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.26_03:41_TAI
===== 475 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.26_10:09_TAI
===== 476 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.26_14:47_TAI
===== 477 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.26_17:40_TAI
===== 478 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.27_02:42_TAI
===== 479 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.27_03:32_TAI
===== 480 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.27_14:06_TAI
===== 481 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.28_08:20_TAI
===== 482 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.28_10:01_TAI
===== 483 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.28_14:33_TAI
===== 484 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.28_16:20_TAI
===== 485 =====
accept NOAA Active Region number 12192 and HARPNUM 4698 at time 2014.10.28_18:50_TAI
===== 486 =====
skip: latitude is out of range for HARPNUM 4698 at time 2014.10.28_21:22_TAI
===== 487 =====
skip: latitude is out of range for HARPNUM 4698 at time 2014.10.29_00:37_TAI
===== 488 =====
skip: latitude is out of range for HARPNUM 4698 at time 2014.10.29_01:35_TAI
===== 489 =====
skip: latitude is out of range for HARPNUM 4698 at time 2014.10.29_04:28_TAI
===== 490 =====
skip: latitude is out of range for HARPNUM 4781 at time 2014.11.05_01:39_TAI
===== 491 =====
accept NOAA Active Region number 12205 and HARPNUM 4781 at time 2014.11.05_22:16_TAI
===== 492 =====
accept NOAA Active Region number 12205 and HARPNUM 4781 at time 2014.11.06_02:49_TAI
===== 493 =====
accept NOAA Active Region number 12205 and HARPNUM 4781 at time 2014.11.06_10:22_TAI
===== 494 =====
accept NOAA Active Region number 12205 and HARPNUM 4781 at time 2014.11.08_15:32_TAI
===== 495 =====
accept NOAA Active Region number 12209 and HARPNUM 4817 at time 2014.11.14_12:03_TAI
===== 496 =====
accept NOAA Active Region number 12209 and HARPNUM 4817 at time 2014.11.15_17:48_TAI
===== 497 =====
accept NOAA Active Region number 12222 and HARPNUM 4874 at time 2014.11.30_06:41_TAI
===== 498 =====
accept NOAA Active Region number 12222 and HARPNUM 4874 at time 2014.12.03_08:10_TAI
===== 499 =====
accept NOAA Active Region number 12222 and HARPNUM 4874 at time 2014.12.03_18:25_TAI
===== 500 =====
accept NOAA Active Region number 12222 and HARPNUM 4874 at time 2014.12.03_19:41_TAI
===== 501 =====
accept NOAA Active Region number 12222 and HARPNUM 4874 at time 2014.12.04_12:25_TAI
===== 502 =====
skip: there are no data for HARPNUM 4941 at time 2014.12.12_05:20_TAI
===== 503 =====
accept NOAA Active Region number 12242 and HARPNUM 4920 at time 2014.12.13_19:33_TAI
===== 504 =====
accept NOAA Active Region number 12242 and HARPNUM 4920 at time 2014.12.16_01:10_TAI
===== 505 =====
accept NOAA Active Region number 12241 and HARPNUM 4941 at time 2014.12.16_19:01_TAI
===== 506 =====
accept NOAA Active Region number 12242 and HARPNUM 4920 at time 2014.12.18_09:44_TAI
===== 507 =====
accept NOAA Active Region number 12242 and HARPNUM 4920 at time 2014.12.20_07:32_TAI
===== 508 =====
accept NOAA Active Region number 12241 and HARPNUM 4941 at time 2014.12.20_12:17_TAI
===== 509 =====
accept NOAA Active Region number 12242 and HARPNUM 4920 at time 2014.12.21_01:49_TAI
===== 510 =====
accept NOAA Active Region number 12249 and HARPNUM 4955 at time 2014.12.26_02:16_TAI
===== 511 =====
accept NOAA Active Region number 12253 and HARPNUM 5011 at time 2015.01.02_09:47_TAI
===== 512 =====
accept NOAA Active Region number 12253 and HARPNUM 5011 at time 2015.01.03_15:36_TAI
===== 513 =====
accept NOAA Active Region number 12257 and HARPNUM 5026 at time 2015.01.12_04:58_TAI
===== 514 =====
skip: latitude is out of range for HARPNUM 5026 at time 2015.01.13_12:58_TAI
===== 515 =====
skip: there are no data for HARPNUM 5107 at time 2015.01.21_04:52_TAI
===== 516 =====
accept NOAA Active Region number 12268 and HARPNUM 5107 at time 2015.01.25_16:53_TAI
===== 517 =====
accept NOAA Active Region number 12268 and HARPNUM 5107 at time 2015.01.27_04:41_TAI
===== 518 =====
skip: latitude is out of range for HARPNUM 5127 at time 2015.01.27_21:37_TAI
===== 519 =====
accept NOAA Active Region number 12268 and HARPNUM 5107 at time 2015.01.28_11:42_TAI
===== 520 =====
accept NOAA Active Region number 12268 and HARPNUM 5107 at time 2015.01.29_00:44_TAI
===== 521 =====
accept NOAA Active Region number 12268 and HARPNUM 5107 at time 2015.01.29_05:36_TAI
===== 522 =====
accept NOAA Active Region number 12277 and HARPNUM 5127 at time 2015.01.29_12:16_TAI
===== 523 =====
accept NOAA Active Region number 12277 and HARPNUM 5127 at time 2015.02.03_02:15_TAI
===== 524 =====
skip: there are no data for HARPNUM 5233 at time 2015.03.01_06:39_TAI
===== 525 =====
skip: there are no data for HARPNUM 5233 at time 2015.03.01_09:48_TAI
===== 526 =====
skip: there are no data for HARPNUM 5233 at time 2015.03.01_09:48_TAI
===== 527 =====
skip: latitude is out of range for HARPNUM 5233 at time 2015.03.01_15:28_TAI
===== 528 =====
skip: latitude is out of range for HARPNUM 5233 at time 2015.03.01_19:31_TAI
===== 529 =====
skip: latitude is out of range for HARPNUM 5233 at time 2015.03.02_01:35_TAI
===== 530 =====
skip: there are no data for HARPNUM 5298 at time 2015.03.04_18:11_TAI
===== 531 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.08_14:33_TAI
===== 532 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.10_00:02_TAI
===== 533 =====
skip: there are no data for HARPNUM 5298 at time 2015.03.10_07:18_TAI
===== 534 =====
skip: there are no data for HARPNUM 5298 at time 2015.03.10_07:57_TAI
===== 535 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.10_18:51_TAI
===== 536 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.11_04:46_TAI
===== 537 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.11_11:50_TAI
===== 538 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.11_12:14_TAI
===== 539 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.11_14:08_TAI
===== 540 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.11_21:51_TAI
===== 541 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.12_04:01_TAI
===== 542 =====
skip: there are no data for HARPNUM 5298 at time 2015.03.12_06:07_TAI
===== 543 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.13_04:40_TAI
===== 544 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.14_09:40_TAI
===== 545 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.14_23:22_TAI
===== 546 =====
accept NOAA Active Region number 12297 and HARPNUM 5298 at time 2015.03.15_10:58_TAI
===== 547 =====
accept NOAA Active Region number 12320 and HARPNUM 5415 at time 2015.04.07_14:43_TAI
===== 548 =====
accept NOAA Active Region number 12322 and HARPNUM 5446 at time 2015.04.20_07:21_TAI
===== 549 =====
skip: there are no data for HARPNUM 1 at time 2015.04.20_10:40_TAI
===== 550 =====
accept NOAA Active Region number 12322 and HARPNUM 5446 at time 2015.04.20_11:57_TAI
===== 551 =====
skip: latitude is out of range for HARPNUM 5446 at time 2015.04.20_15:45_TAI
===== 552 =====
skip: latitude is out of range for HARPNUM 5446 at time 2015.04.20_17:00_TAI
===== 553 =====
skip: latitude is out of range for HARPNUM 5446 at time 2015.04.20_21:45_TAI
===== 554 =====
accept NOAA Active Region number 12325 and HARPNUM 5447 at time 2015.04.20_22:01_TAI
===== 555 =====
skip: latitude is out of range for HARPNUM 5446 at time 2015.04.21_08:44_TAI
===== 556 =====
skip: there are no data for HARPNUM 5446 at time 2015.04.22_10:07_TAI
===== 557 =====
skip: there are no data for HARPNUM 5541 at time 2015.05.04_09:47_TAI
===== 558 =====
skip: there are no data for HARPNUM 5541 at time 2015.05.04_13:53_TAI
===== 559 =====
accept NOAA Active Region number 12335 and HARPNUM 5526 at time 2015.05.04_14:25_TAI
===== 560 =====
accept NOAA Active Region number 12335 and HARPNUM 5526 at time 2015.05.04_17:24_TAI
===== 561 =====
skip: latitude is out of range for HARPNUM 5541 at time 2015.05.05_11:49_TAI
===== 562 =====
skip: there are no data for HARPNUM 5673 at time 2015.06.10_08:55_TAI
===== 563 =====
accept NOAA Active Region number 12360 and HARPNUM 5637 at time 2015.06.12_07:29_TAI
===== 564 =====
skip: latitude is out of range for HARPNUM 5637 at time 2015.06.13_00:59_TAI
===== 565 =====
skip: latitude is out of range for HARPNUM 5653 at time 2015.06.17_01:27_TAI
===== 566 =====
accept NOAA Active Region number 12371 and HARPNUM 5692 at time 2015.06.19_06:48_TAI
===== 567 =====
accept NOAA Active Region number 12371 and HARPNUM 5692 at time 2015.06.20_02:34_TAI
===== 568 =====
accept NOAA Active Region number 12367 and HARPNUM 5673 at time 2015.06.20_09:44_TAI
===== 569 =====
accept NOAA Active Region number 12367 and HARPNUM 5673 at time 2015.06.20_18:20_TAI
===== 570 =====
skip: latitude is out of range for HARPNUM 5738 at time 2015.07.02_12:51_TAI
===== 571 =====
accept NOAA Active Region number 12381 and HARPNUM 5745 at time 2015.07.05_08:44_TAI
===== 572 =====
accept NOAA Active Region number 12381 and HARPNUM 5745 at time 2015.07.05_20:40_TAI
===== 573 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.20_20:34_TAI
===== 574 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.21_06:49_TAI
===== 575 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.21_13:23_TAI
===== 576 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.21_21:24_TAI
===== 577 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.23_07:33_TAI
===== 578 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.23_17:46_TAI
===== 579 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.26_05:44_TAI
===== 580 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.27_13:16_TAI
===== 581 =====
accept NOAA Active Region number 12403 and HARPNUM 5885 at time 2015.08.27_19:03_TAI
===== 582 =====
skip: latitude is out of range for HARPNUM 5885 at time 2015.08.29_03:30_TAI
===== 583 =====
accept NOAA Active Region number 12415 and HARPNUM 5956 at time 2015.09.16_09:40_TAI
===== 584 =====
skip: there are no data for HARPNUM 5974 at time 2015.09.19_05:03_TAI
===== 585 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.26_10:40_TAI
===== 586 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.26_21:00_TAI
===== 587 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.27_07:35_TAI
===== 588 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.27_13:18_TAI
===== 589 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.27_14:58_TAI
===== 590 =====
accept NOAA Active Region number 12423 and HARPNUM 5982 at time 2015.09.28_03:16_TAI
===== 591 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.28_03:43_TAI
===== 592 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.28_05:16_TAI
===== 593 =====
skip: latitude is out of range for HARPNUM 5982 at time 2015.09.28_05:37_TAI
===== 594 =====
skip: there are no data for HARPNUM 5983 at time 2015.09.28_05:56_TAI
===== 595 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.28_06:43_TAI
===== 596 =====
skip: latitude is out of range for HARPNUM 5982 at time 2015.09.28_08:51_TAI
===== 597 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.28_11:15_TAI
===== 598 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.28_19:24_TAI
===== 599 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.29_10:59_TAI
===== 600 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.29_13:20_TAI
===== 601 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.09.30_13:10_TAI
===== 602 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.10.01_00:13_TAI
===== 603 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.10.01_12:26_TAI
===== 604 =====
accept NOAA Active Region number 12422 and HARPNUM 5983 at time 2015.10.01_17:18_TAI
===== 605 =====
skip: latitude is out of range for HARPNUM 5983 at time 2015.10.03_02:41_TAI
===== 606 =====
skip: latitude is out of range for HARPNUM 6015 at time 2015.10.14_23:31_TAI
===== 607 =====
skip: there are no data for HARPNUM 6015 at time 2015.10.15_06:16_TAI
===== 608 =====
skip: latitude is out of range for HARPNUM 6026 at time 2015.10.16_20:23_TAI
===== 609 =====
skip: latitude is out of range for HARPNUM 6026 at time 2015.10.16_20:42_TAI
===== 610 =====
accept NOAA Active Region number 12443 and HARPNUM 6063 at time 2015.10.30_17:52_TAI
===== 611 =====
accept NOAA Active Region number 12445 and HARPNUM 6052 at time 2015.11.03_12:03_TAI
===== 612 =====
accept NOAA Active Region number 12449 and HARPNUM 6078 at time 2015.11.08_13:12_TAI
===== 613 =====
skip: there are no data for HARPNUM 6206 at time 2015.12.21_03:34_TAI
===== 614 =====
accept NOAA Active Region number 12473 and HARPNUM 6206 at time 2015.12.23_02:12_TAI
===== 615 =====
accept NOAA Active Region number 12497 and HARPNUM 6327 at time 2016.02.11_10:47_TAI
===== 616 =====
accept NOAA Active Region number 12497 and HARPNUM 6327 at time 2016.02.12_15:24_TAI
===== 617 =====
accept NOAA Active Region number 12497 and HARPNUM 6327 at time 2016.02.13_19:26_TAI
===== 618 =====
accept NOAA Active Region number 12497 and HARPNUM 6327 at time 2016.02.14_11:00_TAI
===== 619 =====
accept NOAA Active Region number 12567 and HARPNUM 6670 at time 2016.07.20_00:46_TAI
===== 620 =====
accept NOAA Active Region number 12567 and HARPNUM 6670 at time 2016.07.20_01:49_TAI
===== 621 =====
accept NOAA Active Region number 12567 and HARPNUM 6670 at time 2016.07.22_02:11_TAI
===== 622 =====
accept NOAA Active Region number 12567 and HARPNUM 6670 at time 2016.07.22_05:16_TAI
===== 623 =====
accept NOAA Active Region number 12567 and HARPNUM 6670 at time 2016.07.22_05:31_TAI
===== 624 =====
skip: latitude is out of range for HARPNUM 6670 at time 2016.07.23_06:20_TAI
===== 625 =====
skip: latitude is out of range for HARPNUM 6670 at time 2016.07.23_17:43_TAI
===== 626 =====
skip: there are no data for HARPNUM 1 at time 2016.08.06_14:44_TAI
===== 627 =====
accept NOAA Active Region number 12615 and HARPNUM 6870 at time 2016.11.28_17:23_TAI
===== 628 =====
accept NOAA Active Region number 12615 and HARPNUM 6870 at time 2016.11.28_23:38_TAI
===== 629 =====
accept NOAA Active Region number 12644 and HARPNUM 6972 at time 2017.04.01_13:00_TAI
===== 630 =====
accept NOAA Active Region number 12644 and HARPNUM 6972 at time 2017.04.01_20:33_TAI
===== 631 =====
skip: there are no data for HARPNUM 1 at time 2017.07.02_16:15_TAI
===== 632 =====
accept NOAA Active Region number 12665 and HARPNUM 7075 at time 2017.07.08_03:18_TAI
===== 633 =====
skip: there are no data for HARPNUM 7110 at time 2017.08.19_01:52_TAI
===== 634 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.03_05:49_TAI
===== 635 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.03_19:37_TAI
===== 636 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.03_20:02_TAI
===== 637 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.03_22:14_TAI
===== 638 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.04_01:08_TAI
===== 639 =====
skip: there are some missing keywords for HARPNUM 7115 at time 2017.09.04_03:51_TAI
===== 640 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.04_04:53_TAI
===== 641 =====
skip: there are no data for HARPNUM 7115 at time 2017.09.04_06:40_TAI
===== 642 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.04_17:43_TAI
===== 643 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.05_09:10_TAI
===== 644 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.05_15:56_TAI
===== 645 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.05_19:30_TAI
===== 646 =====
skip: there are no data for HARPNUM 1 at time 2017.09.05_23:39_TAI
===== 647 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.06_05:02_TAI
===== 648 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.06_09:54_TAI
===== 649 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.07_02:24_TAI
===== 650 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.07_15:47_TAI
===== 651 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.07_23:45_TAI
===== 652 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.08_04:28_TAI
===== 653 =====
accept NOAA Active Region number 12673 and HARPNUM 7115 at time 2017.09.08_11:04_TAI
===== 654 =====
skip: latitude is out of range for HARPNUM 7115 at time 2017.09.08_23:53_TAI
Here is the number of events associated with the negative class:
no_CME_data = negative_result[0]
negative_class = negative_result[1]
print("There are", len(no_CME_data), "no-CME events in the negative class.")
There are 430 no-CME events in the negative class.
Step 3: Feature selection¶
Some of the features within a data set may be powerful for distinguishing between the positive and negative class, whereas others may be redundant or irrelevant. To identify features in the former category, we use a univariate feature selection method, which is implemented in the feature selection module of the scikit-learn library, for feature scoring.
To improve the performance of the feature selection algorithm, we’ll normalize each feature so that they lie within similar ranges. To do this, we subtract from every feature its median value and divide by its standard deviation.
CME_data = np.array(CME_data)
no_CME_data = np.array(no_CME_data)
def normalize_the_data(flare_data):
flare_data = np.array(flare_data)
n_elements = flare_data.shape[0]
for j in range(flare_data.shape[1]):
standard_deviation_of_this_feature = np.std(flare_data[:, j])
median_of_this_feature = np.median(flare_data[:, j])
for i in range(n_elements):
flare_data[i, j] = (
flare_data[i, j] - median_of_this_feature) / (standard_deviation_of_this_feature)
return flare_data
no_CME_data = normalize_the_data(no_CME_data)
CME_data = normalize_the_data(CME_data)
print("There are", no_CME_data.shape[0], "flares with no associated CMEs.")
print("There are", CME_data.shape[0], "flares with associated CMEs.")
There are 430 flares with no associated CMEs.
There are 77 flares with associated CMEs.
Let’s look at the distribution of one feature for the active regions that both flared and produced a CME (green) and for the active regions that flared but did not produce a CME (red). You can change the value of i
in the code block below to see that some features are totally useless as there is barely any difference in the distributions for the positive and negative class. As such, we can throw such features out of our sample. It’s a good idea to do some feature selection before running the SVM, so as to reduce noise (in this case, with only 18 features, there’s not too much noise to begin with).
sharps = ['Total unsigned flux', 'Mean gradient of total field',
'Mean current helicity (Bz contribution)', 'Mean photospheric magnetic free energy',
'Fraction of Area with Shear > 45 deg', 'Total unsigned current helicity',
'Mean gradient of horizontal field', 'Mean characteristic twist parameter, alpha',
'Mean angle of field from radial', 'Mean gradient of vertical field',
'Mean vertical current density', 'Total unsigned vertical current',
'Sum of the modulus of the net current per polarity',
'Total photospheric magnetic free energy density', 'Mean shear angle',
'Area of strong field pixels in the active region', 'Sum of flux near polarity inversion line',
'Absolute value of the net current helicity']
i = 2
# For the positive class (green)
mu_fl = np.mean(CME_data[:, i])
sigma_fl = np.std(CME_data[:, i])
num_bins = 15
n_fl, bins_fl, patches_fl = plt.hist(
CME_data[:, i], num_bins, normed=1, facecolor='green', alpha=0.5)
y_fl = scipy.stats.norm.pdf(bins_fl, mu_fl, sigma_fl)
plt.plot(bins_fl, y_fl, 'g--', label='positive class')
# For the negative class (red)
mu_nofl = np.mean(no_CME_data[:, i])
sigma_nofl = np.std(no_CME_data[:, i])
n_nofl, bins_nofl, patches_nofl = plt.hist(
no_CME_data[:, i], num_bins, normed=1, facecolor='red', alpha=0.5)
y_nofl = scipy.stats.norm.pdf(bins_nofl, mu_nofl, sigma_nofl)
plt.plot(bins_nofl, y_nofl, 'r--', label='negative class')
text_style = dict(fontsize=16, fontdict={'family': 'monospace'})
plt.xlabel('Normalized '+sharps[i], **text_style)
plt.ylabel('Number (normalized)', labelpad=20, **text_style)
fig = plt.gcf()
fig.set_size_inches(10, 5)
fig.savefig('fscore_tmp.png', bbox_inches='tight')
legend = plt.legend(loc='upper right', fontsize=12, framealpha=0.0, title='')
legend.get_frame().set_linewidth(0.0)
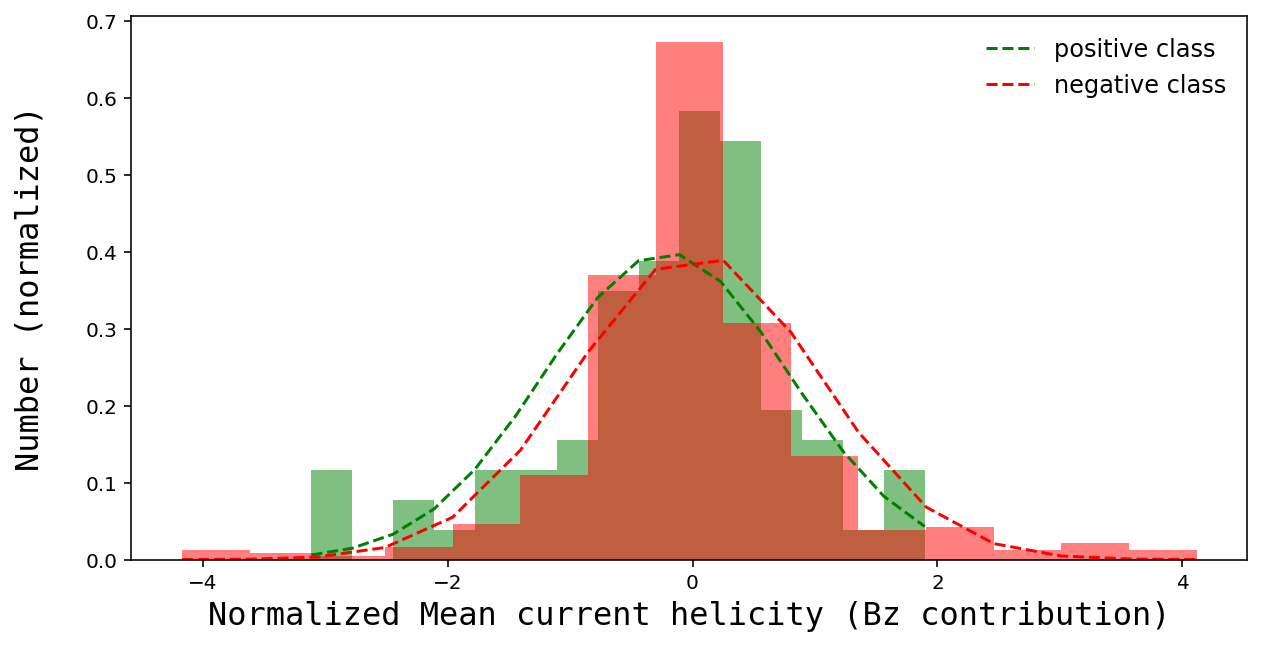
Now we will compute the Univariate F-score for feature selection. It is a very simple method: the F-score measures the distance between the two distributions for a given feature (inter-class distance), divided by the sum of the variances for this feature (intra-class distance). We can use the sklearn.feature_selection
module to do this:
# import the feature selection method
from sklearn.feature_selection import SelectKBest, f_classif
# select the number of features
N_features = 18
Nfl = CME_data.shape[0]
Nnofl = no_CME_data.shape[0]
yfl = np.ones(Nfl)
ynofl = np.zeros(Nnofl)
# k is the number of features
selector = SelectKBest(f_classif, k=N_features)
selector.fit(np.concatenate((CME_data, no_CME_data), axis=0),
np.concatenate((yfl, ynofl), axis=0))
scores = selector.scores_
print(scores)
[0.23129349 1.66767301 3.85850986 2.26629803 0.05521982 0.02133073
0.97811712 2.89386483 0.40696658 0.00615498 0.6648416 0.05306211
0.20062115 0.04774681 0.33766216 0.06349227 1.18890519 0.00428464]
It’s not easy to interpret the scores in this fashion, so let’s plot the results. The higher the Univariate Fisher Score, the more predictive the feature.
plt.clf()
order = np.argsort(scores)
orderedsharps = [sharps[i] for i in order]
y_pos2 = np.arange(18)
plt.barh(y_pos2, sorted(scores/np.max(scores)))
plt.ylim((-1, 19))
plt.yticks(y_pos2, orderedsharps, fontsize=12)
plt.xlabel('Normalized Fisher Score', fontsize=15)
plt.title('Normalized Univariate Fisher Score Per Feature', fontsize=15)
plt.subplots_adjust(left=0.5, right=1.0)
fig = plt.gcf()
fig.set_size_inches(9, 5)
plt.show()
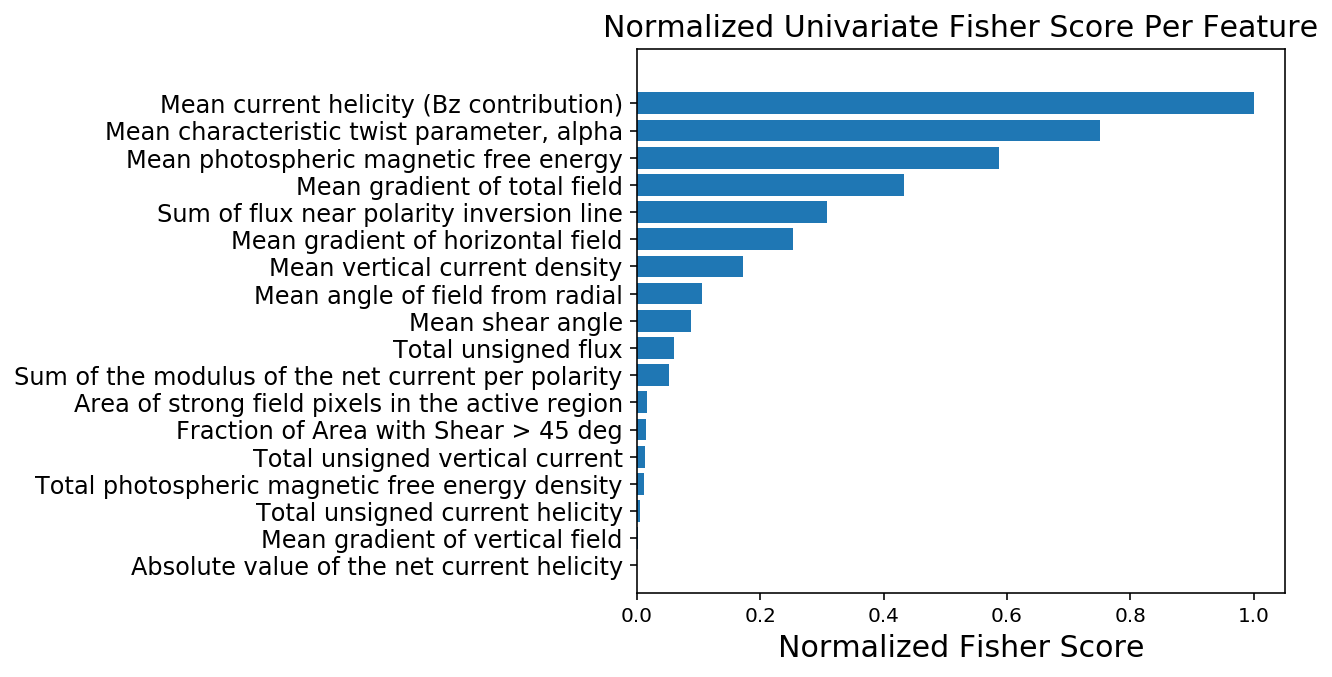
Step 4: The support vector machine¶
Now we initialize the support vector machine on the data. The SVM uses non-linear decision functions to map the feature space into a higher-dimensional space, where the positive and negative examples can be separated linearly by a hyperplane.
This is incredibly non-intuitive. But we can think of a simpler example. Suppose we had two classes: CME-producing and non-CME producing active regions. And suppose we had two features: the total flux in these regions, and the total area of these regions. We could construct a two-dimentional feature space, where we plot the flux against the area of each active region. Positive examples could be indicated by an X and negatives ones by an O. In theory, if our data behaved well, we could draw a line between these classess.
Since we have 18 features, the SVM constructs an 18-dimensional feature space. In this feature space, the decision boundary separating the positive and negative examples may be non-linear. As such, the algorithm then enlarges this 18-dimensional feature space (using the function indicated by the kernel
parameter in the svm.SVC
function) into a higher-dimensional feature space wherein it is possible to linearly separate the positive and negatives classes. There are lots of people trying to work on how to visualize these multi-dimensional feature spaces, which is an active area of research.
number_of_examples = Nfl + Nnofl
C = 4.0
gamma = 0.075
class_weight = {1: 6.5}
clf = svm.SVC(C=C, gamma=gamma, kernel='rbf', class_weight=class_weight,
cache_size=500, max_iter=-1, shrinking=True, tol=1e-8, probability=True)
Step 5: Stratified k-folds cross-validation¶
Now we run and evaluate the performance of the SVM. There are lots of different ways to evaluate the performance of a classifier, which we discuss in Section 4 of Bobra & Couvidat (2015). We’re going to choose a metric called the True Skill Score, or the TSS, which we can calculate from four quantities: true positives, true negatives, false positives, and false negatives. We prefer the TSS to all the other metrics as it is insensitive to the class imbalance ratio and thus best for comparison to other groups. The TSS is symmetrically distributed about 0: i.e., it goes from [-1, 1] where 0 represents no skill and a negative value represents a perverse prediction. Thus we are able to predict CMEs in a fashion better than randomly guessing. Here we define a confusion table to measure the performance of our binary classification:
def confusion_table(pred, labels):
"""
computes the number of TP, TN, FP, FN events given the arrays with predictions and true labels
and returns the true skill score
Args:
pred: np array with predictions (1 for flare, 0 for nonflare)
labels: np array with true labels (1 for flare, 0 for nonflare)
Returns: true negative, false positive, true positive, false negative
"""
Nobs = len(pred)
TN = 0.
TP = 0.
FP = 0.
FN = 0.
for i in range(Nobs):
if (pred[i] == 0 and labels[i] == 0):
TN += 1
elif (pred[i] == 1 and labels[i] == 0):
FP += 1
elif (pred[i] == 1 and labels[i] == 1):
TP += 1
elif (pred[i] == 0 and labels[i] == 1):
FN += 1
else:
print("Error! Observation could not be classified.")
return TN, FP, TP, FN
Now we run the SVM on our data and cross-validate our results. In our case, the positive sample size is quite small (both objectively and compared to the negative sample size). Therefore, we use a stratified k-folds cross-validation method, which makes k partitions of the data set and uses k-1 folds for training the SVM and 1 fold for testing the trained SVM. The stratification preserves the ratio of positive to negative examples per fold. Then we can permute over the partitions such that each partition eventually makes its way into the testing set. For each individual testing set, we can calculate a skill score. Then we can average the skill scores over the total number of testing sets.
To compute the TSS, we must first select a value of k. k can be arbitrarily defined and take any value between 2 and number_of_examples
, so we can explore this parameter space. As k approaches number_of_examples
, the k-fold method reduces to the Leave One Out method, in which only one example is in the testing set and all other examples are in the training set. The literature suggests this method is not the best, so we can stray away from high values of k. Many studies (e.g. Kohavi, 1995) recommend the stratified 10-fold cross-validation to reduce variance and bias. Here, we test their recommendation by computing the TSS using 50 k values, ranging from 2 to 52.
# lists to hold the TSS and standard deviation of the TSS
array_of_avg_TSS = np.ndarray([50])
array_of_std_TSS = np.ndarray([50])
# xdata are the examples
# ydata are the labels
xdata = np.concatenate((CME_data, no_CME_data), axis=0)
ydata = np.concatenate((np.ones(Nfl), np.zeros(Nnofl)), axis=0)
# mdata contain metadata about the active region that will be useful
# when we interpret the results using LIME
mdata = np.concatenate((positive_class, negative_class), axis=0)
# compute the TSS for a variety of k ranging from 2 to 52
# this is to see how the TSS varies as a function of k, and to test if k=10 really makes sense
for k in range(2, 52):
skf = StratifiedKFold(n_splits=k, shuffle=True)
these_TSS_for_this_k = []
for train_index, test_index in skf.split(xdata, ydata):
# xtrain are the examples in the training set
xtrain = xdata[train_index]
# ytrain are the labels in the training set
ytrain = ydata[train_index]
# xtest are the examples in the testing set
xtest = xdata[test_index]
ytest = ydata[test_index] # ytest are the labels in the testing set
# metadata useful for interpreting with LIME
mtrain = mdata[train_index]
# metadata useful for interpreting with LIME
mtest = mdata[test_index]
clf.fit(xtrain, ytrain)
TN, FP, TP, FN = confusion_table(clf.predict(xtest), ytest)
if (((TP+FN) == 0.0) or (FP+TN) == 0.0):
these_TSS_for_this_k.append(np.nan)
continue
else:
these_TSS_for_this_k.append(TP/(TP+FN) - FP/(FP+TN))
TSS_k = np.array(these_TSS_for_this_k)
array_of_avg_TSS[k-2] = np.mean(TSS_k)
array_of_std_TSS[k-2] = np.std(TSS_k)
Now we can plot the mean TSS per k, using the standard deviation as the error in the TSS. We see that for high values of k, the standard deviation in the TSS can be greater than the mean. These points are indicated in blue.
fig, ax = plt.subplots(figsize=(10, 8)) # define the size of the figure
orangered = (1.0, 0.27, 0, 1.0) # create an orange-red color
cornblue = (0.39, 0.58, 0.93, 1.0) # create a cornflower-blue color
# define some style elements
marker_style_red = dict(linestyle='', markersize=8,
fillstyle='full', color=orangered, markeredgecolor=orangered)
marker_style_blue = dict(linestyle='', markersize=8,
fillstyle='full', color=cornblue, markeredgecolor=cornblue)
text_style = dict(fontsize=16, fontdict={'family': 'monospace'})
# ascribe the data to the axes
k = np.arange(50)+2
for i in range(50):
if (array_of_avg_TSS[i] > array_of_std_TSS[i]):
ax.errorbar(k[i], array_of_avg_TSS[i],
yerr=array_of_std_TSS[i], linestyle='', color=orangered)
ax.plot(k[i], array_of_avg_TSS[i], 'o', **marker_style_red)
if (array_of_avg_TSS[i] <= array_of_std_TSS[i]):
ax.errorbar(k[i], array_of_avg_TSS[i],
yerr=array_of_std_TSS[i], linestyle='', color=cornblue)
ax.plot(k[i], array_of_avg_TSS[i], 'o', **marker_style_blue)
# set plot limits
plt.xlim(xmax=52, xmin=0)
plt.ylim(ymax=1.1, ymin=0)
# label the axes and the plot
ax.set_xlabel('k', **text_style)
ax.set_ylabel('TSS', labelpad=20, **text_style)
plt.title(r'TSS per k using stratified k-fold cross-validation', **text_style)
fig = plt.gcf()
fig.set_size_inches(10, 5)
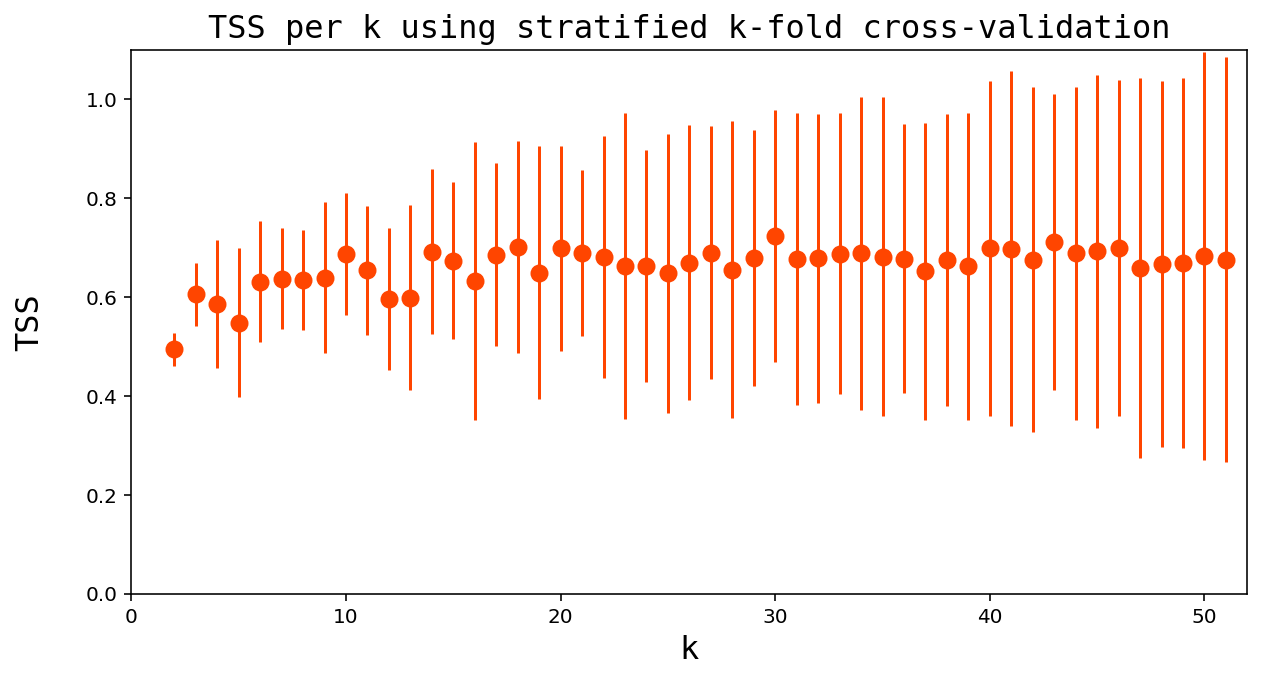
As such, we confirm that high k-values result in a high variance. We find it reasonable to use the stratified 10-fold cross-validation method to compute the TSS and will follow this recommendation. Therefore we report this score as our final result:
print("The TSS equals", array_of_avg_TSS[9],
"plus or minus", array_of_std_TSS[9], ".")
The TSS equals 0.6547952047952048 plus or minus 0.13053813744235288 .
Addendum : Local Interpretable Model-Agnostic Explanations (LIME)¶
Machine-learning is a powerful technique that can help us predict CMEs. However, our goal is not only to predict CMEs, but also to quantitatively understand which signatures indicate the imminent eruption of a CME. But the practical successes of machine-learning algorithms are often not matched by successes in understanding, and this has become an issue within the machine-learning community (Rahimi and Recht, 2017).
The SVM is a good model to start with, because it is (relatively) simple and we can use the Univariate Fisher Score to identify the most predictive features. But it would also be useful to figure out why each individual active region was classed as positive or negative. To do this, we can use a tool called LIME (or Local Interpretable Model-Agnostic Explanations).
First, we initialize the LIME explainer:
explainer = lime.lime_tabular.LimeTabularExplainer(
xtrain, feature_names=sharps, class_names=['CME', 'no CME'], discretize_continuous=True)
Then we use the explainer to explain its choice for a particular active region. To do this, the LIME module generates neighborhood data by randomly perturbing the values of the features associated with this active region. If, for any given feature, this perturbation does not change the outcome of the prediction, this feature isn’t useful along the perturbed dimension. If, for any given feature, the perturbation does change the outcome of the prediction, this feature is useful along the perturbed dimension. Thus the explainer can determine which features are useful under which conditions.
i = np.random.randint(0, xtest.shape[0])
exp = explainer.explain_instance(xtest[i], clf.predict_proba, num_features=8)
Now we can visualize the results. The bottom left panel shows the probabilities assigned to this particular example (which are computed by the SVM via the probability=True
parameter). The right panel plots the weights per feature (and indicates the values of these weights at the end of each horizontal bar). The text describes the conditions under which this feature is predictive.
print("Here is the prediction explanation for NOAA Active Region",
mtest[i][1], "(HARPNUM ", mtest[i][0], "),\n which produced a", mtest[i][2], "class flare on", mtest[i][3], ".")
exp.show_in_notebook(show_table=False, show_all=False)
Here is the prediction explanation for NOAA Active Region 11884 (HARPNUM 3321 ),
which produced a M1.6 class flare on 2013.11.01_22:21_TAI .
Here is the same information in words:
explained_list = exp.as_list()
for i in range(len(explained_list)):
if (explained_list[i][1]) < 0:
feature_sign = 'no CME'
else:
feature_sign = 'CME'
print("The following condition:", explained_list[i][0], "\n predicts",
feature_sign, "with a model weight of", abs(explained_list[i][1]), ".")
The following condition: Mean angle of field from radial > 0.77
predicts no CME with a model weight of 0.1834177544201487 .
The following condition: -0.33 < Absolute value of the net current helicity <= 0.00
predicts CME with a model weight of 0.07848095237996201 .
The following condition: Mean current helicity (Bz contribution) > 0.41
predicts no CME with a model weight of 0.06556078879003055 .
The following condition: -0.00 < Mean gradient of horizontal field <= 0.76
predicts no CME with a model weight of 0.06293982511197757 .
The following condition: -0.01 < Mean shear angle <= 0.66
predicts CME with a model weight of 0.05550865167166992 .
The following condition: -0.36 < Total unsigned flux <= -0.00
predicts CME with a model weight of 0.05389626441321625 .
The following condition: 0.00 < Mean gradient of total field <= 0.65
predicts CME with a model weight of 0.03938864824472328 .
The following condition: -0.02 < Fraction of Area with Shear > 45 deg <= 0.70
predicts CME with a model weight of 0.025936027814020466 .